Posted by Deanna Garcia and Louis Wasserman - Software Developers, and Developer Advocate, James Ward At Google, we’re investing deeply in the Kotlin language and ecosystem. Android development is now Kotlin first, our engineering teams work on language evolution through the Kotlin Foundation, and inside of Google we’re using Kotlin more and more to build our backend services. We love Kotlin for its expressiveness, safety, simple async support through coroutines, and easy bidirectional interoperability with the Java programming language. Last year, we open sourced Kotlin support for gRPC, the open source Remote Procedure Call (RPC) framework that powers thousands of microservices at Google. We’re excited to deepen our investment in the Kotlin language with official support for Kotlin in the open source Protocol Buffers project (a.k.a. “protos”), Google’s platform-neutral, high-performance data interchange format. From a proto definition, you can use the new built-in Kotlin support in the proto compiler to generate idiomatic Kotlin Domain Specific Languages (DSLs). For example, here’s a simple protocol buffer message representing a series of dice rolls: In the Java language, constructing a series of dice rolls might look like this: With this release, protos offer an expressive set of DSL factory methods that make this code elegant and idiomatic in Kotlin. Here is the equivalent dice roll code written using the new Kotlin proto bindings: The Kotlin version uses Kotlin type-safe builders, which makes it concise and removes the need to explicitly call a Note: Since protos use the The new Kotlin Protos work great with gRPC Kotlin providing a concise syntax for messages and services. Let's walk through a basic sample. Here is a basic "Greeter" gRPC service proto: Check out the full example proto source. The non-blocking server can be implemented concisely, taking advantage of the Kotlin proto builders: Check out the full example server source. The non-blocking client also can take advantage of the type-safe Kotlin builders with concise syntax: Check out the full example client source. Those code examples really illustrate how concise the syntax is, which means less boilerplate to write and less for our brains to parse as we try to read the code. What isn't evident in these examples is how nice the experience is when writing high-performance RPC code. Thanks to static typing and the type-safe builders, you can easily code-complete your way through code that is "correct" in-that the types / properties are consistent with the protos. The protobuf compiler (protoc) now has built-in support for generating Kotlin code. A bit of configuration is needed to tell your build tool (Maven, Gradle, etc) to do that. For Gradle builds it looks like: Check out the full example Gradle build. Give the complete gRPC Kotlin example a spin and also explore other aspects like the Android client and native client (built with GraalVM Native Image) which both use the lite protos. For an example Maven project check out the grpc-hello-world-mvn sample. For examples that can be deployed with a couple clicks on Google Cloud Run (a fully managed serverless platform) check out: grpc-hello-world-gradle, grpc-hello-world-streaming (server push), or grpc-hello-world-bidi-streaming (bi-directional streaming). To learn more about Kotlin protos check out these docs: Let us know how it goes and if you have any Kotlin proto issues by filing them within the official protobuf project. Additional thanks to Adam Cozzette, Brent Shaffer, David Jones, David Winer, Jeff Grimes, John Pampuch, and Kevin Bierhoff for their contributions to this release!Google’s commitment to Kotlin
Kotlin for protocol buffers
message DiceSeries {
message DiceRoll {
int32 value = 1; // value of this roll, e.g. 2..12
string nickname = 2; // string nickname, e.g. "snake eyes"
}
repeated DiceRoll rolls = 1;
}DiceSeries series = DiceSeries.newBuilder()
.addRoll(DiceRoll.newBuilder()
.setValue(5))
.addRoll(DiceRoll.newBuilder()
.setValue(20)
.setNickname("critical hit"))
.build()val series = diceSeries {
rolls = listOf(
diceRoll { value = 5 },
diceRoll {
value = 20
nickname = "critical hit"
}
)
}build
method. Note that this works with both the proto compiler’s standard and "proto lite" modes, the latter generating smaller, higher performance classes which are more suitable for Android. get
prefix for their fields and Kotlin recognizes that as a property, reading from a proto already works smoothly from Kotlin as if the proto were a data class. val totalRolls = series.rolls.map { it.value }.sum() // 5 + 20 = 25
Kotlin Protos and gRPC Kotlin
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}class HelloWorldService : GreeterCoroutineImplBase() {
override suspend fun sayHello(request: HelloRequest) = helloReply {
message = "hello, ${request.name}"
}
}val stub = GreeterCoroutineStub(channel)
val request = helloRequest { name = "world" }
val response = stub.sayHello(request)
println("Received: ${response.message}")protobuf {
// omitted protoc and plugins config
generateProtoTasks {
all().forEach {
// omitted plugins config
it.builtins {
id("kotlin")
}
}
}
}Learn More
Source: Google Developers Blog
Author Archives: Google Developers
Easily connect Google Pay with your preferred payment processor
Posted by Stephen McDonald, Developer Relations Engineer, Google Pay
Easily connect Google Pay with your preferred payment processor
Adding Google Pay as a payment method to your website or Android application provides a secure and fast checkout option for your users. To enable Google Pay, you will first need a Payment Service Provider (PSP). For the integration this means understanding how your payments processing stack works with Google Pay APIs.
End-to-end PSP samples
To make integration easier, we’ve launched a new open source project containing end-to-end samples for a range of PSPs, demonstrating the entire integration process - from client-side configuration, to server-side integration with the PSPs, using their respective APIs and client libraries where applicable. The project uses Node.js and is written in JavaScript, which most developers should find familiar. Each of the samples in the project are implemented in a consistent fashion, and demonstrate best practices for integrating Google Pay and your preferred PSP with your website or Android application.
A recent study by 451 Research showed that for merchants with over 50% of sales occurring online, 69% of merchants used multiple PSPs. With these new samples, we demonstrate how you can implement an entirely consistent interface to multiple PSPs, streamlining your codebase while also providing more flexibility for the future.
Lastly, we've also added support to both the Web and Android Google Pay sample applications, making it easy to demonstrate the new PSP samples. Simply run the PSP samples project, and configure the Web or Android samples to send their cart information and Google Pay token to the PSP samples app, which will then send the relevant data to the PSP's API and return the PSP's response back.
Initial PSPs
To start with, we've included support for 6 popular PSPs: Adyen, Braintree, Checkout.com, Cybersource, Square, and Stripe. But that's just the beginning. If you're involved with a PSP that isn't yet included, we've made adding new PSPs to the open source project as simple as possible. Just head on over to the GitHub repository which contains instructions on contributing your preferred PSP to the project.
Launching Google Pay for your website
When you’ve completed your testing, submit your website integration in the Google Pay Business Console. You will need to provide your website’s URL and screenshots to complete the submission.
Summing it up
Integrating Google Pay into your website is a great way to increase conversions and to improve the purchasing experience for your customers, and with these new open source samples, the process is even easier.
What do you think? Follow us on Twitter for the latest updates @GooglePayDevs
Do you have any questions? Let us know in the comments below or tweet using #AskGooglePayDevs.
Source: Google Developers Blog
#IamaGDE: Martin Kleppe
#IamaGDE series presents: Google Maps Platform
The Google Developers Experts program is a global network of highly experienced technology experts, influencers, and thought leaders who actively support developers, companies, and tech communities by speaking at events and publishing content.
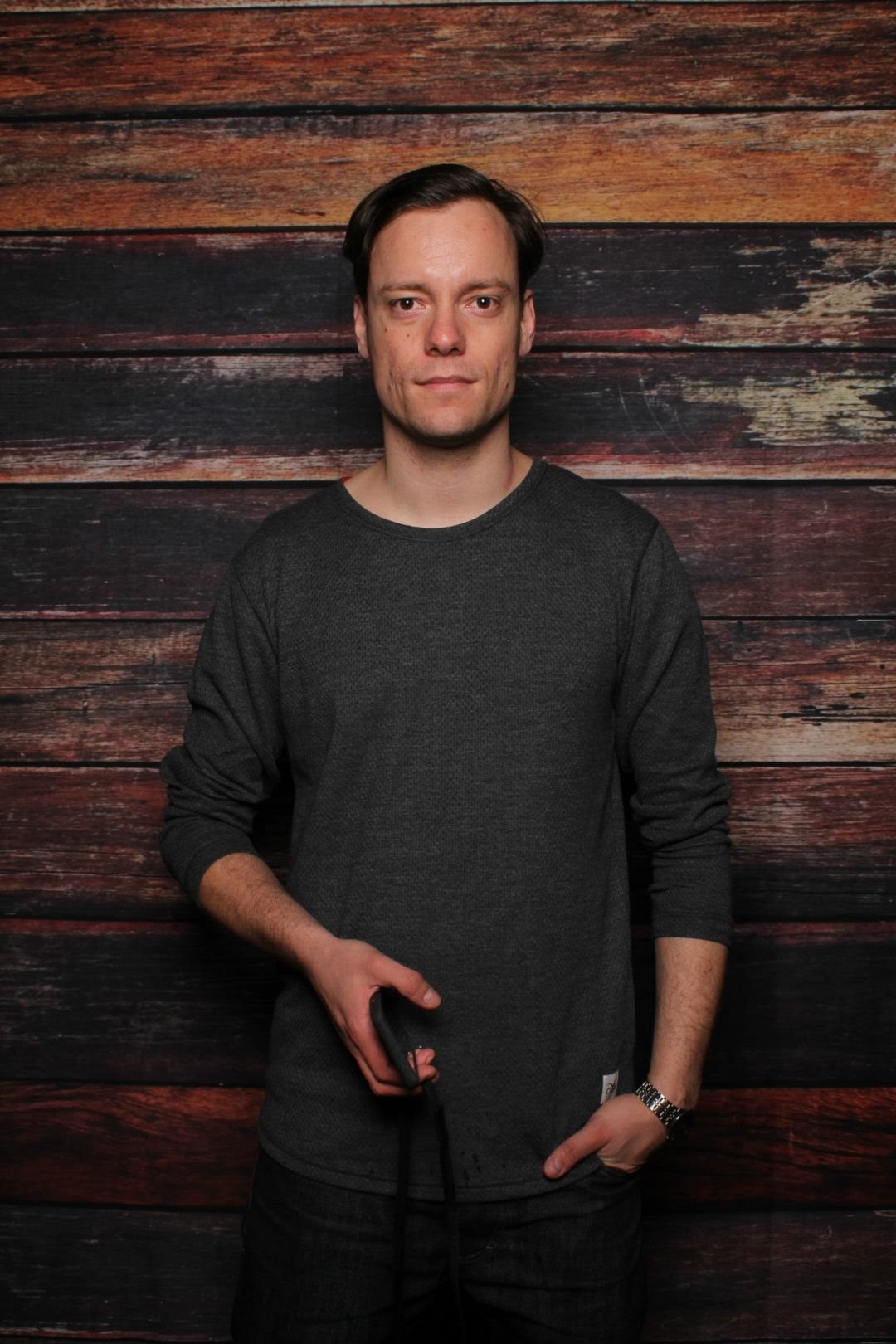
Martin Kleppe is a co-founder of Ubilabs, a Google Cloud partner since 2012. He is the head of development, responsible for R&D and technical strategy. He talks with customers to understand their challenges and which location technologies will best serve them. He also works with partners like Google to determine the best ways to combine the latest technologies.
Ubilabs – a data and location technology consultancy specializing in product and software development, visualization, implementation, and data management – built the official feature tour and travel app demos for Google Maps Platform at Google I/O 2021.
“We worked with the latest Google Maps Platform APIs to create demos that showcase the combined features,” he says.
Ubilabs also works closely with the Google Earth team, developing content and UI components for its storytelling platform. In addition, they are in close contact with the Google Maps Platform team, giving feedback on the latest products and features before they’re released.
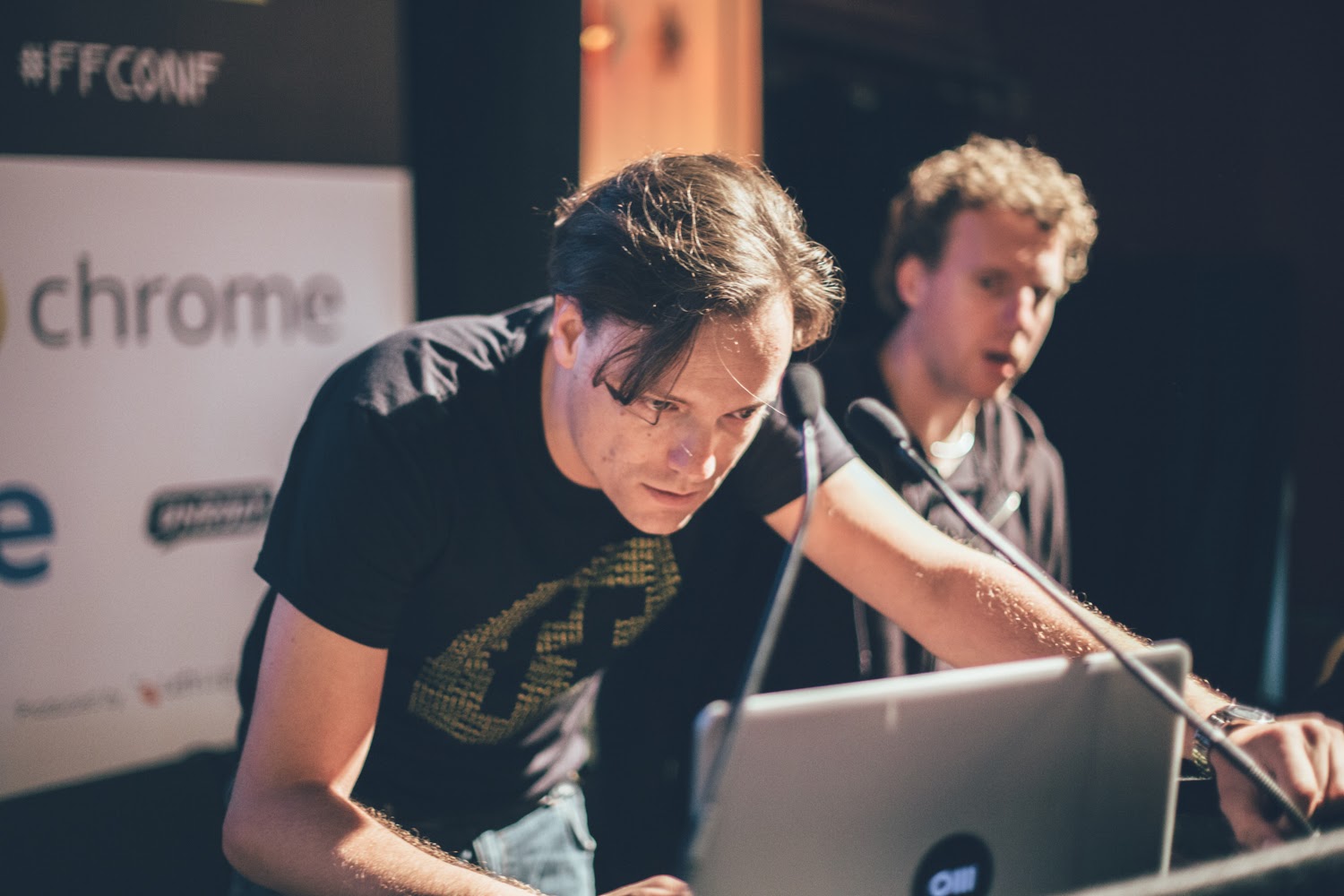
Kleppe’s background is in design and coding web applications. At Bauhaus University, Kleppe was in the Media Art and Design program, where he learned the principle of simplicity in design, with a focus on what users want. Students’ projects combined technology and art, and while programming courses weren’t required, students were expected to explain their thinking. Kleppe worked on live blogging platforms using maps and mobile phones, even before popular maps APIs and iPhones came out.
“I was always focused on interactive maps,” Kleppe says. “Before Google Maps, I used NASA satellite imagery and Japanese cell phones with access to the user’s location. That’s how I got into programming and into maps.”
After university, he decided to start a company.
“When the Google Maps API came out, I thought it was a perfect time to make a business out of it,” he said. “We started with a few friends, and then the company took off.”
Kleppe keeps his focus on the value of maps to users: Maps connect virtual users on computer screens to the real world, providing a connection between humans and data, and they provide context around a location.
“Maps, especially Google Maps, make it easy for users to get access to information”, says Kleppe. “With the Google Maps API, it was super easy to put things on a map.”
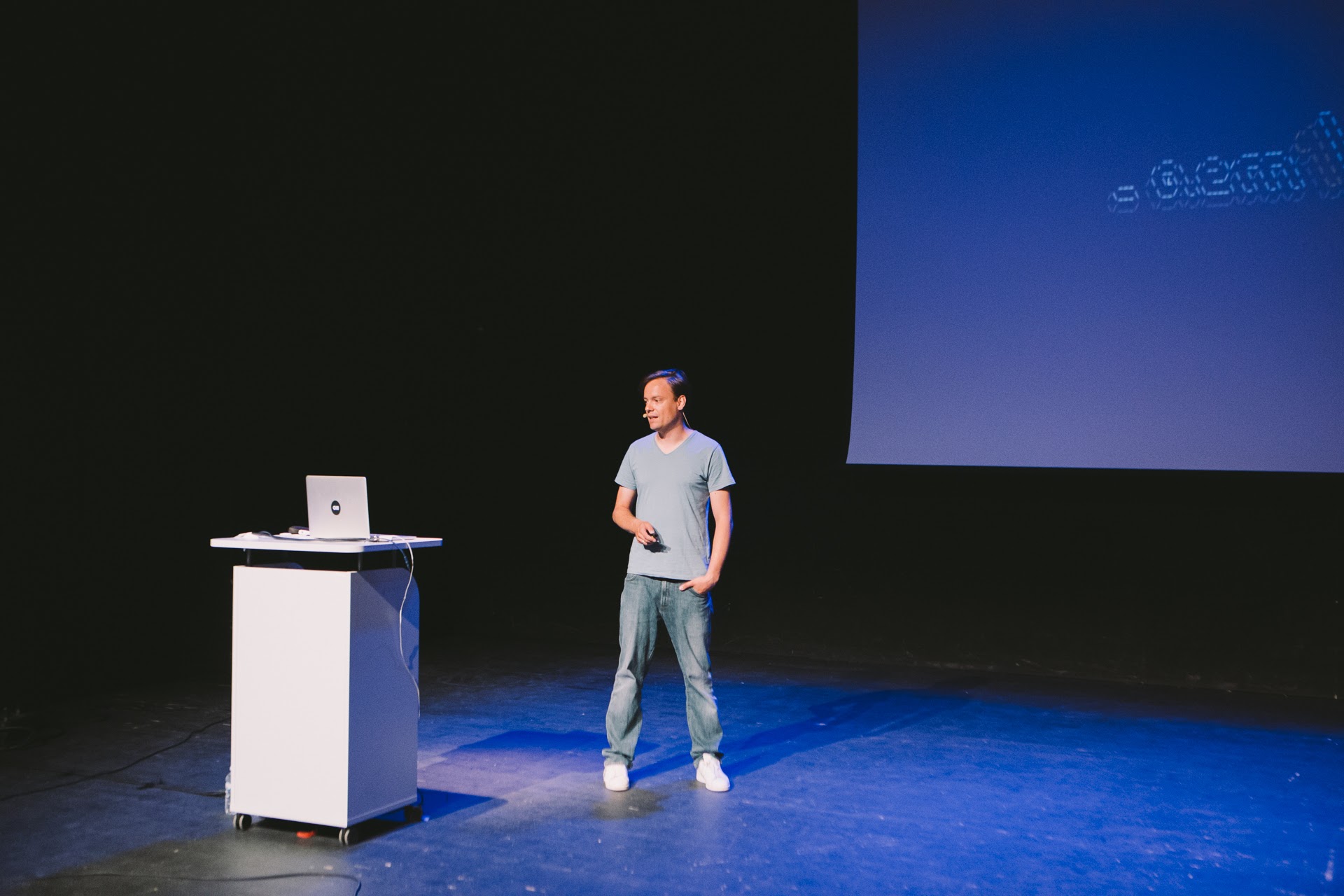
Getting involved in the developer community
Two different events got Kleppe involved in the developer community. In Hamburg, where he lives, he belonged to a small local JavaScript user group and gave frequent talks that led to group discussions about the Google Maps API and the usability aspects of the Google API. Ubilabs was also invited to talk about Google Maps at a Google technical event. The Google office in Hamburg held different meetups, and Kleppe found himself giving 12 talks a year and organizing conferences with tracks on topics including JavaScript and Google Maps.
When Kleppe’s first child was born, he cut down on his travel and poured his energy into his burgeoning relationships with developer advocates and teams. Around the same time, Kleppe became a Google Developer Expert (GDE).
“I wanted to become a GDE because I’m always eager to share Ubilabs’ latest use of Google technologies and inspire people with showcases of our work, pushing the limit of what’s possible,” Kleppe says. “As a GDE, you’re closer to the development and what’s happening at Google.”
GDEs get previews of APIs, know the people who are working on projects at Google, and give feedback.
“It is very interesting and important for us as a company to be prepared and to know what clients will be able to use in the future,” he says. “And it’s important for me, as well, because I always want to learn the latest things and be on top of tech trends.”
Kleppe enjoys interacting with the other Google Maps Platform GDEs, who live all over the world.
“They are teachers at universities, self-employed developers who work on small apps, and full-time speakers and educators,” Kleppe says. “That’s valuable to me because I work with clients, and I have my own views, but seeing how other people are working on the same thing from other perspectives helps me understand why different aspects of APIs exist.”
These days, Kleppe primarily works with the members of the Google Maps Platform, Google Earth, and Google Cloud product teams who develop new APIs, to provide his expert feedback.
“We work with a lot of different clients and understand what developers, clients, and users need and can pass useful feedback,” he says. “I think there is value to the community in having us test and review the new APIs. We think about new ways to use them, and if we find errors, we can help the Google Maps Platform team address these issues before the APIs are released to the public.”
In 2020, Kleppe found himself even more connected with the Google team when Ubilabs was selected to build the official Google Maps Platform demos for Google I/O 2021, featuring the new WebGL-powered features that are currently in beta release.
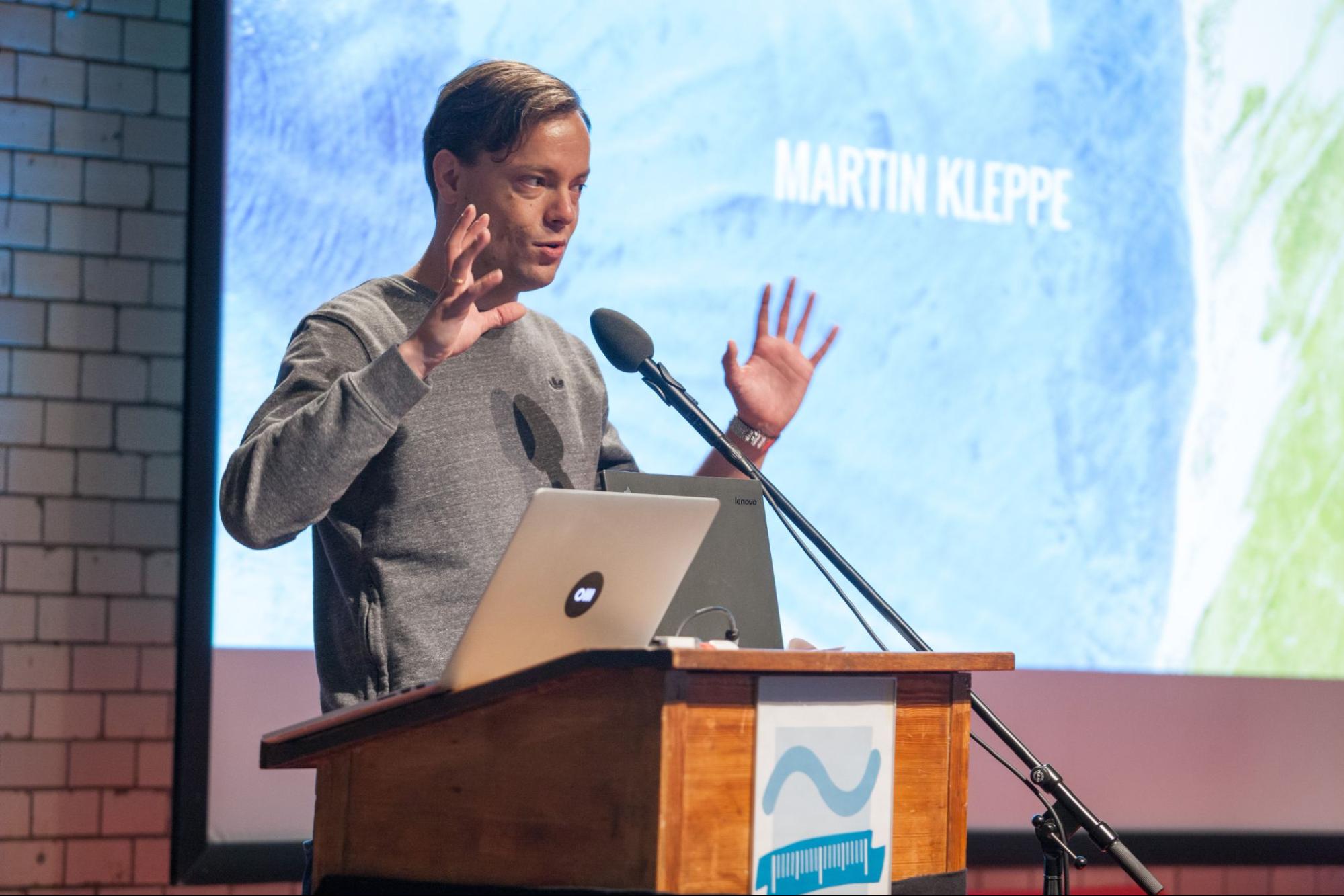
Favorite Maps features and current projects
“WebGL is a different way of rendering maps,” says Kleppe. “The underlying technology has huge potential. It uses GPU-accelerated computing, where you use the graphics card in your machine to render 3D buildings and place 3D objects in space. Before this technology was available, your data was an additional layer that covered the map. Now, you have control over how the data is visually merged with the existing map. This will provide the user an immersive experience, like looking at a view of a city.”
Kleppe notes that the Google Maps APIs used to be limited to about a thousand objects, but now it can handle millions of moving points on a map. For him, being able to visualize and analyze large amounts of data is useful to Ubilabs because the company focuses on data visualization and analysis.
“You can visualize, animate, and draw data on the map,” he says. “You can analyze real-time information on maps.”
Kleppe says the best and most interesting feature of the Google Maps Platform APIs is the ability to combine so many data sources – the base map with streets, a layer to draw business info, an extra API to calculate directions, and the ability to draw one’s own information on top of all that.
“You’re not limited to the set of data in the API,” he says. “You’re always free to merge your own data into an abstract view of the world.”
Ubilabs put 3D features on their wish list years ago.
“The 3D feature is the most awaited feature for us,” he says. “Nine years later, it’s finally becoming a reality, so I’m super excited.”
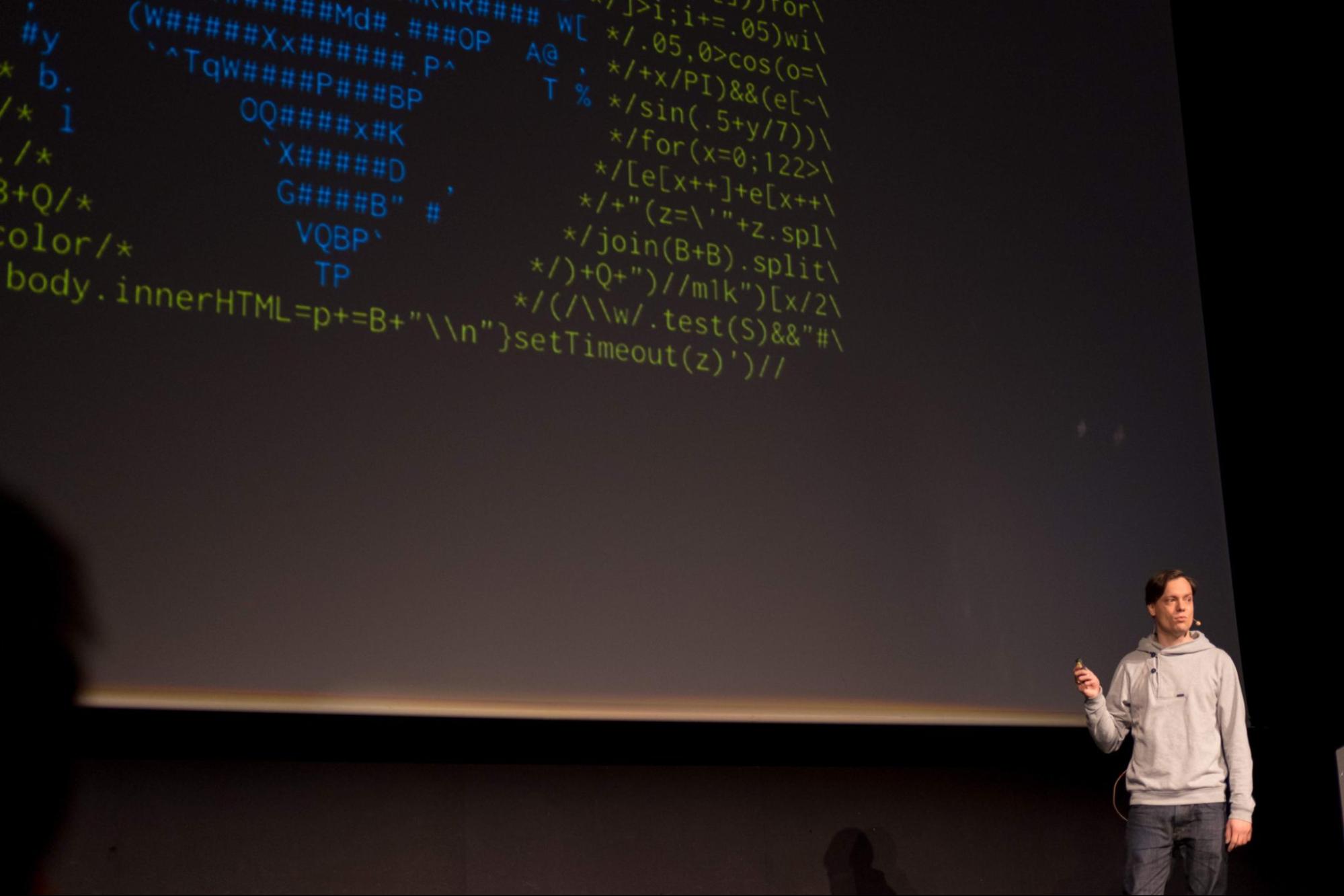
Future plans
In his role at Ubilabs, Kleppe is sharpening the company’s strategic goals and adding focus on the field of data analytics with location aspects.
“We can work with clients and enable them to gain insights from their data,” he says. Every dataset has a connection to something in the real world, and if you use that information, you can make decisions about your business.”
His GDE role has allowed Kleppe to try Google Cloud’s data-specific products.
“We combine the visualization power of maps with the power of data analytics in Google Cloud and stream massive amounts of highly dynamic location data; for example, to track ships or analyze movements of assets,” Kleppe says. “We built a cloud team with a clear focus on data analytics on GCP the past three years, to look into how to preprocess data in the cloud, and we see a lot of potential.”
Follow Martin on Twitter at @aemkei | Read more about Ubilabs | Check out Martin’s experiments.
For more information on Google Maps Platform, visit our website or learn more about our GDE program.
Source: Google Developers Blog
#IamaGDE: Bhavesh Bhatt
Welcome to #IamaGDE - a series of spotlights presenting Google Developer Experts (GDEs) from across the globe. Discover their stories, passions, and highlights of their community work.
Bhavesh Bhatt is a data scientist and a YouTuber based in Mumbai, India. He began his career as an engineer and transitioned to machine learning and data science. Now, he trains other data scientists to be successful in their careers. Bhavesh is known for his popular YouTube videos and an open source app he created to help Indian citizens locate vaccination appointments near them in the spring of 2021. He has been a GDE since 2019.
Meet Bhavesh Bhatt, Google Developer Expert in Machine Learning and Data Science.
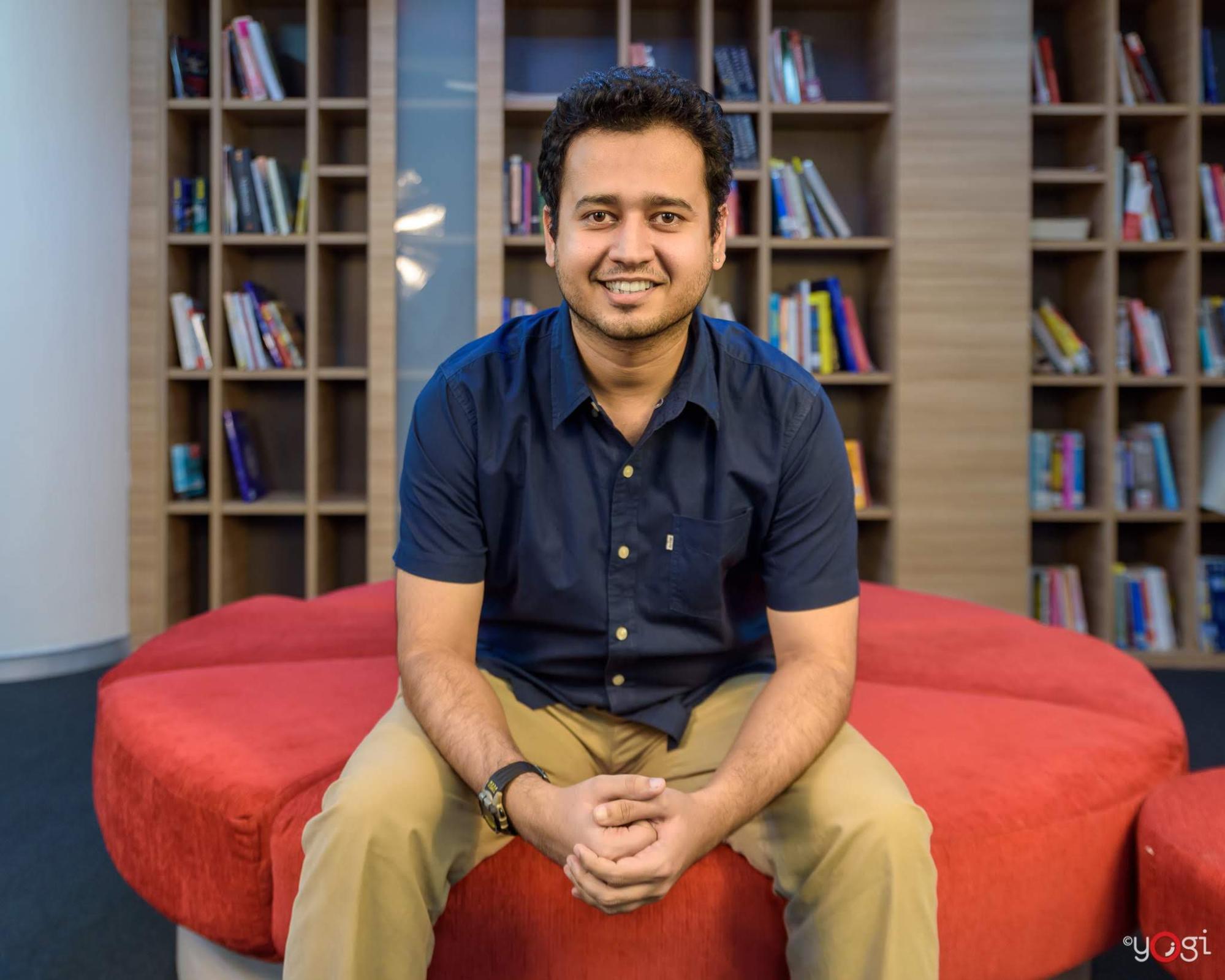
The Early Days
Bhavesh Bhatt has always been technical and has always enjoyed experimenting with coding circuits and creating end-to-end solutions. He earned his Bachelor of Engineering (BE) degree in 2013 from the University of Mumbai and his Master's degree in 2016 from BITS Pilani K K Birla Goa Campus in Embedded Systems. He began his career in the Engineering Development Group at MathWorks and later worked as a software developer at Cisco Systems.
Bhavesh took a course in machine learning in Mumbai to move his career toward machine learning, and he started the next phase of his career as a data scientist at FlexiLoans. There, his role involved automating processes using deep learning to decrease the TAT of loan applications. Bhavesh has worked on object detection, object classification, and OCR-related solutions and is currently a data scientist at Fractal Analytics.
“It’s been an interesting journey so far in data science and machine learning,” he says.
In 2017, he started creating videos on machine learning and data science, as a way to contribute what he had learned to the developer community. He enjoyed creating end-to-end videos and sharing them for free on YouTube, and his subscriber base kept growing. He now has about 33,000 subscribers to his channel and hundreds of videos posted there.
In August 2018, he became an instructor at Greyatom and has helped aspiring data scientists make a successful career transition ever since. He has worked with several EdTech startups to develop their machine learning course curriculum and has reviewed multiple machine learning books.
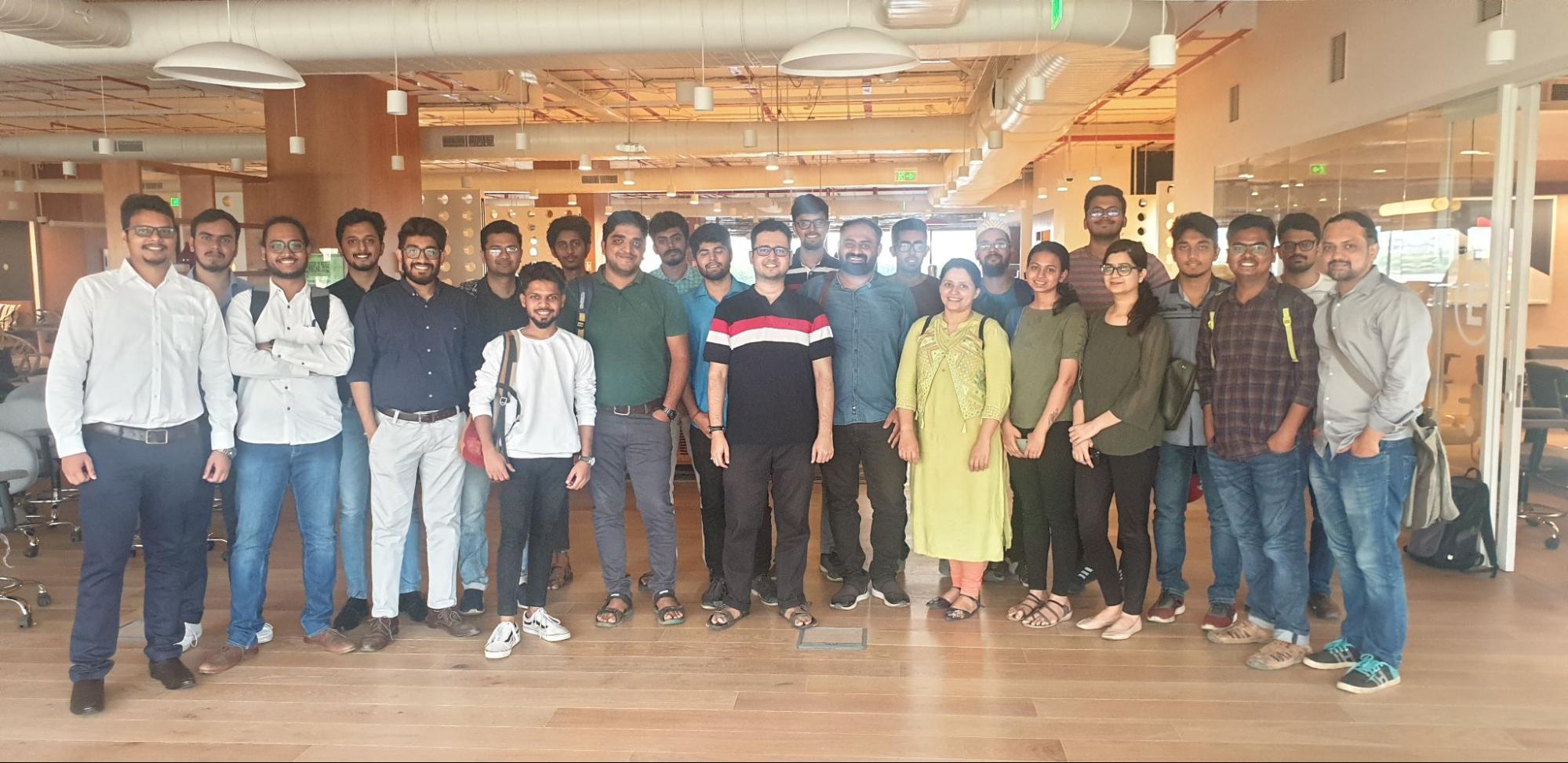
Becoming a GDE
Bhavesh has been a GDE since October 2019.
“I have had an exponential learning curve, all thanks to the Google developer expert group,” he says. “The Google developer expert program has helped me grow in terms of understanding different technologies, like Tensorflow, and Google Cloud.”
He appreciates having access to Google’s cutting-edge work on machine learning as a GDE.
“Google is in the forefront in driving research and natural language processing,” he says. “We get to interact with Googlers and get insights on research.”
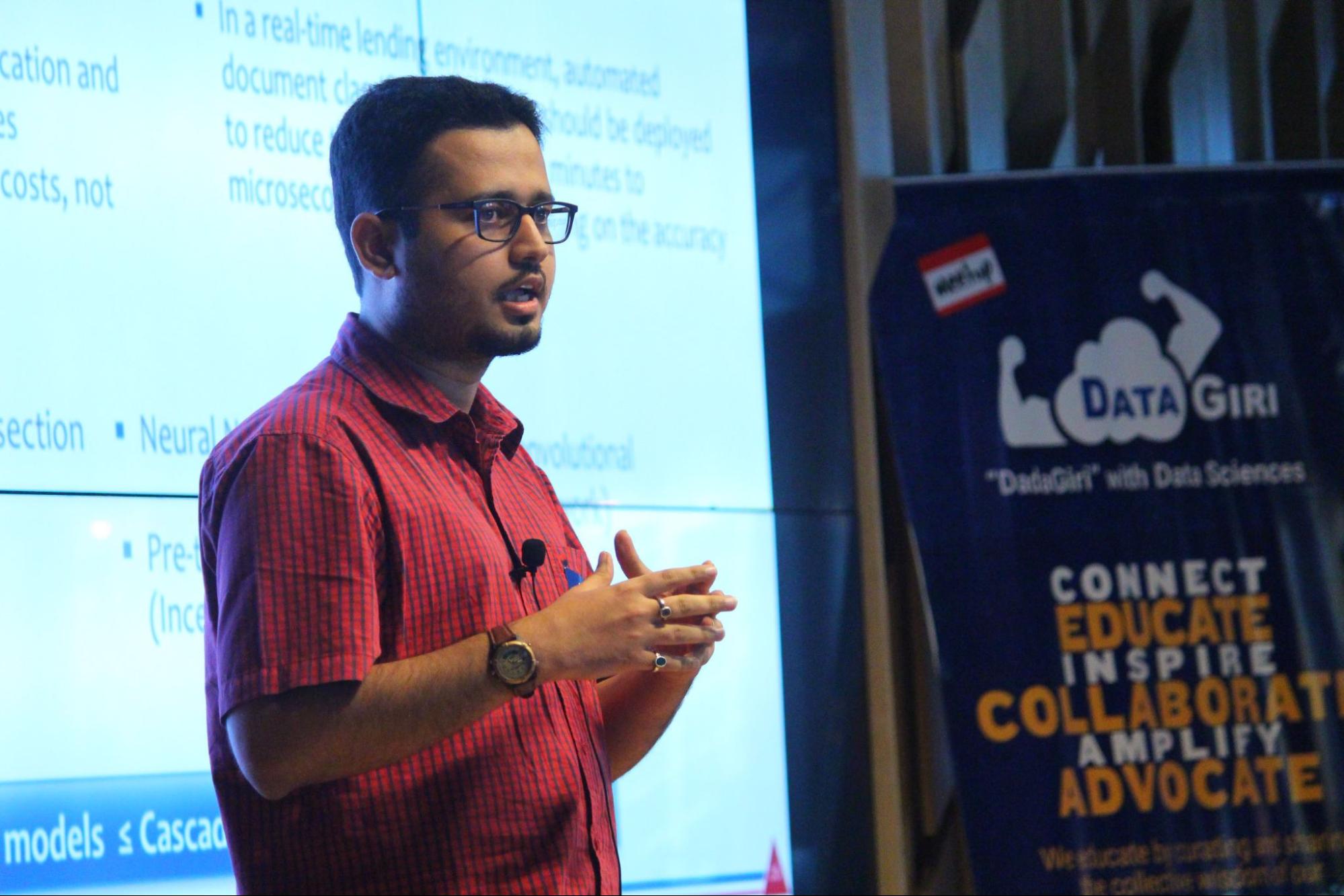
Building a Vaccination Availability Dashboard
India’s dire second wave of COVID-19 infections took place in April and May of 2021. At the same time, the country had rolled out its vaccination program, and demand for vaccination appointments was high. People were trying to book appointments through a single portal that Bhavesh felt could be optimized to handle the high volume of traffic it was receiving.
“While exploring the vaccination website, I found that there was an API that developers could access,” says Bhavesh.
He played around with the API to understand its structure and how to extract data from it, as well as what types of data he could extract. He explored the different end points that were in the API. Then, he created an early version of a dashboard that pulled data from the server and displayed it in real time, allowing people to track the available vaccination slots near them.
“I created the first version of this application using a very popular programming language called S-Python,” Bhavesh says. “I used Google software called Google Colaboratory in order to create the initial solution.”
Google Colaboratory, or Colab for short, allows developers to write and execute Python in the browser, requiring no configuration and providing free access to GPUs and easy sharing. Data scientists can use Colab to analyze and visualize data using popular Python libraries. Colab is also useful for machine learning: Developers can import an image dataset, train an image classifier on it, and evaluate the model in a few lines of code. Bhavesh used it extensively for his project..
“I don’t code on a local machine; I prefer Google Colab because of the flexibility of reading and understanding one cell at a time,” he says. “I added a layer of protection to only add IP addresses from India, and then I had to move the dashboard to my local machine.”
Bhavesh tried scaling his solution in a local script that could run on his machine, but he knew that to roll it out to a larger audience, he’d have to deploy it elsewhere. He had limited knowledge of Google Cloud, so he reached out to the India GDE ecosystem and got connected to a Cloud GDE who hosted the web application on an Indian server, so people could use it. Once the prototype was deployed on Google Cloud, Bhavesh created a YouTube video to reach out as many developers and users as possible, so people could start utilizing it.
“People used it to its fullest potential,” Bhavesh says. “Lots of people used it to book slots and get vaccinated.”
Other developers created enhanced versions of Bhavesh’s script.
“I was just displaying the slots available at the nearest vaccination center, but others created solutions based on mine, where they’d send availability notifications on Signal, WhatsApp, and Telegram,” he says. “Overall, the application was received really well by the community, and a lot of people reached out to me and thanked me for creating it.”
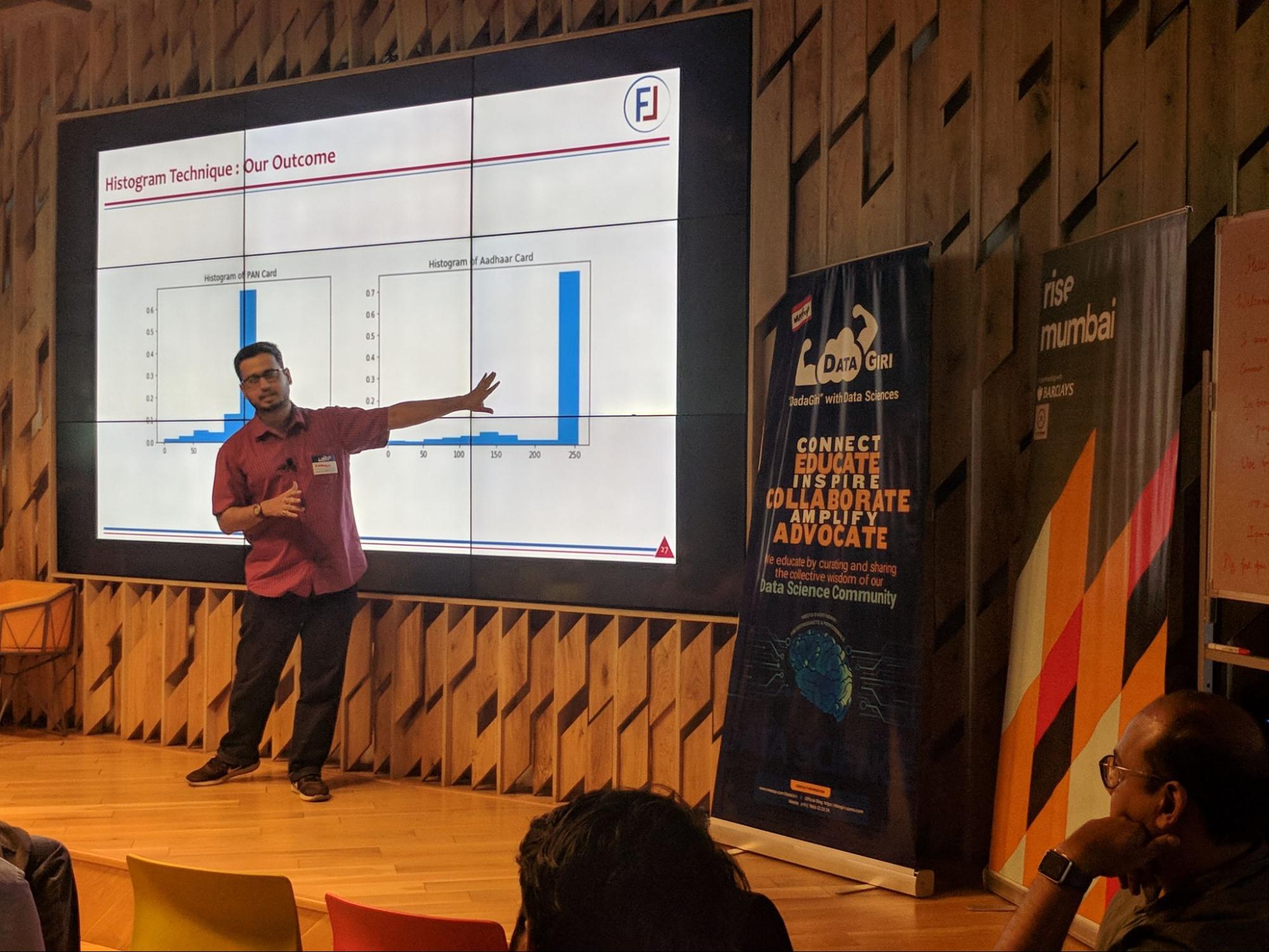
Bhavesh’s advice to fellow developers
Bhavesh recommends that developers explore as many technologies as possible, including software, engineering, data science, and machine learning. He also encourages developers to teach others, by writing blogs and tutorials or by posting videos.
“If you have learned something new, share it with the community,” he advises. “It will help the community and is a good opportunity for individual growth, as well.”
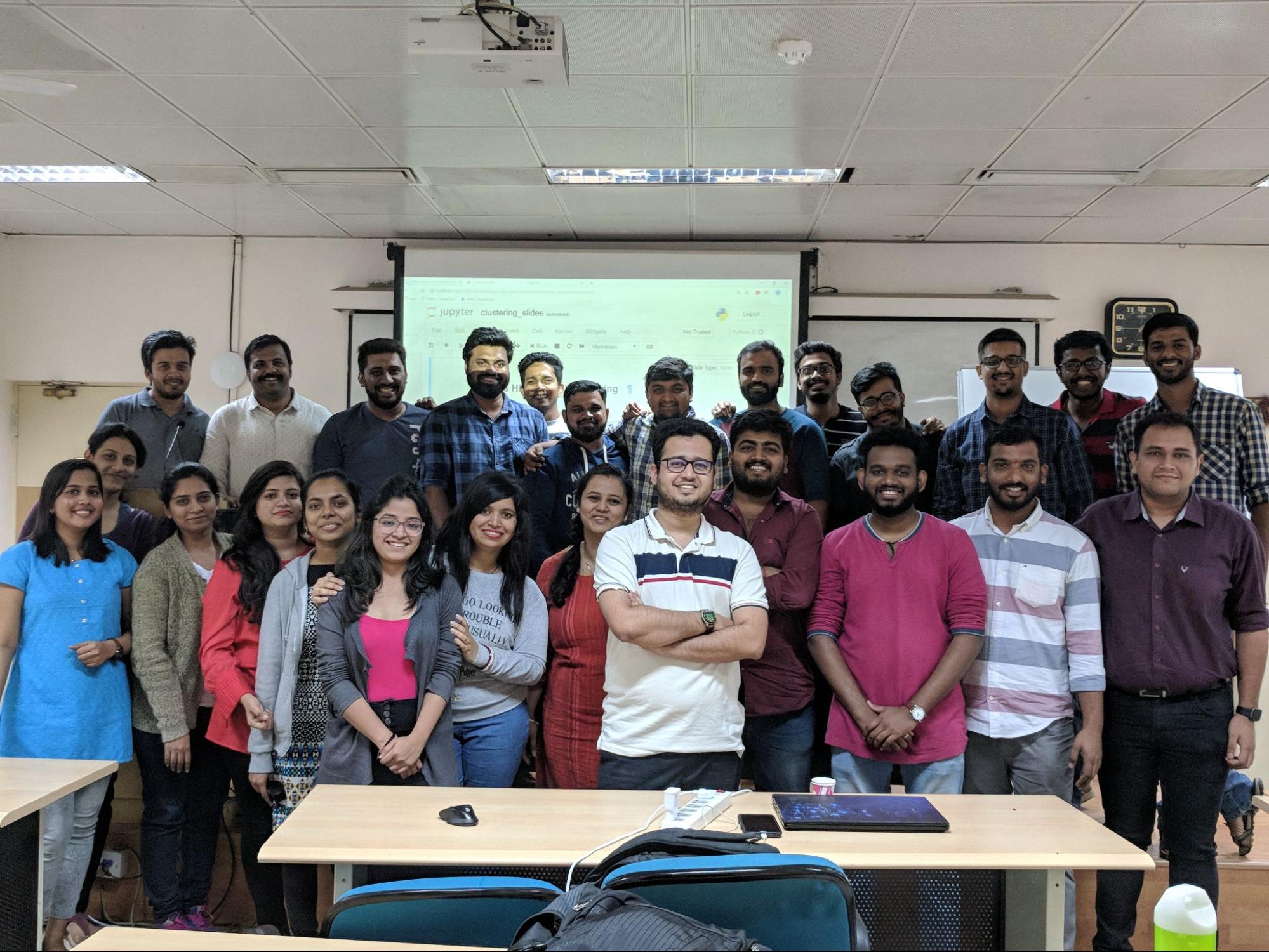
Watch more on Bhavesh and his story here!
Source: Google Developers Blog
How a Developer Student Lead Increased Representation in Campus Clubs Through Community
Posted by Kyle Paul, Google Developer Student Club Regional Lead CA & US
When Chloe Quijano arrived at the University of Toronto to study Applied Sciences, she immediately noticed that few of the professors or teaching assistants in the program looked like her. She noticed a lack of relatable role models online and on social media as well.
Chloe felt strongly about the importance of representation. Whether based on race, gender, ethnicity, sexual orientation or disability status, she understood the importance of being able to find relatable role models in her field--and the implications of a lack of diversity. "It holds back the students wishing to go into the field," she says.
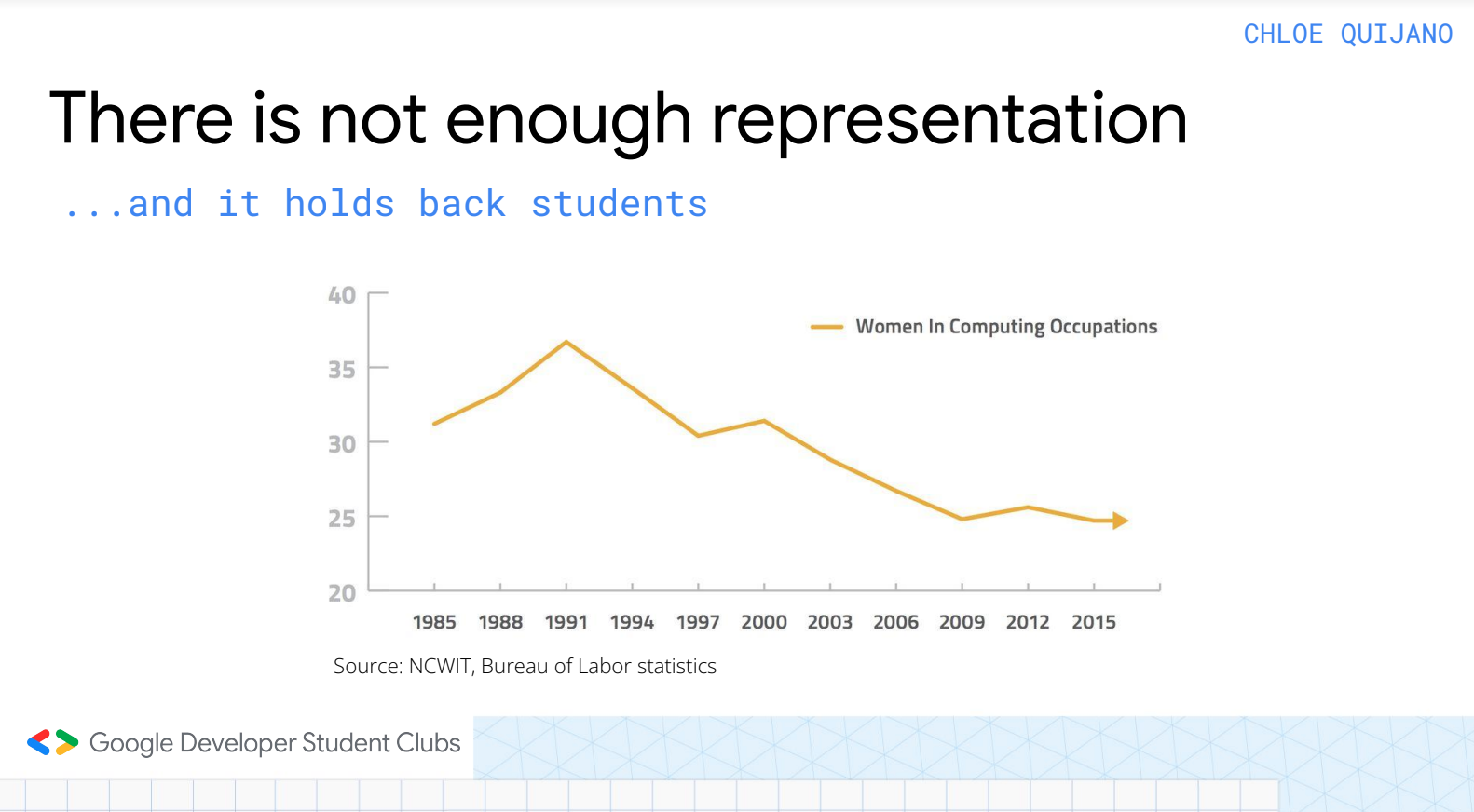
Bureau of Labor Statistics findings on women in computing occupations
Worse, she points out, those that do enter technical careers may experience impostor syndrome: “We often downplay the skills we have as developers.”
Discovering Google Developer Student Clubs
Data backs up Chloe’s impression about the lack of diversity in tech: According to the National Center for Women & Information Technology (NCWIT), women made up only a quarter of the workforce in computing in 2020. Of those, just 3% were African-American, 7% Asian, and 2% Hispanic.
Determined to find a way to combat these trends, Chloe decided to look for a supportive community where she could help make a difference. While searching for a technology club where she could meet and learn from others, she stumbled across the university’s Google Developer Student Club. Noticing that the club was soliciting applications to serve as a lead, she decided to apply—and got the post!
Chloe quickly took steps to advance the group’s mission: to empower all students in technology. To help students like her connect with leaders and role models from a diverse range of backgrounds and experiences, she spearheaded the effort to organize the first-ever DSC Women in Tech Conference.
Held last March, the two-day virtual event featured 13 speakers from a range of backgrounds—including CEOs, Google employees, and recent graduates who landed internships and positions at prominent tech companies. Topics included charting your own path in tech, landing that first job, going the entrepreneurship route, and leveraging the power of a personal narrative. Hands-on workshops included a day-long hackathon challenge focused on redesigning a website to be more accessible and user-friendly for senior citizens. Ultimately, the conference drew more than 250 attendees from around the world, including South Korea, Morocco, Brazil, and China.
Expanding the Range of GDSC Events
After the conference’s success, Chloe continued organizing initiatives and programs for her GDSC group. She helped start a monthly tech meetup for women students, and a weekly online series highlighting potential careers paths, and featuring tech leaders as guests. In just under eight months, the chapter hosted 40 events, reaching more than 1700 participants.
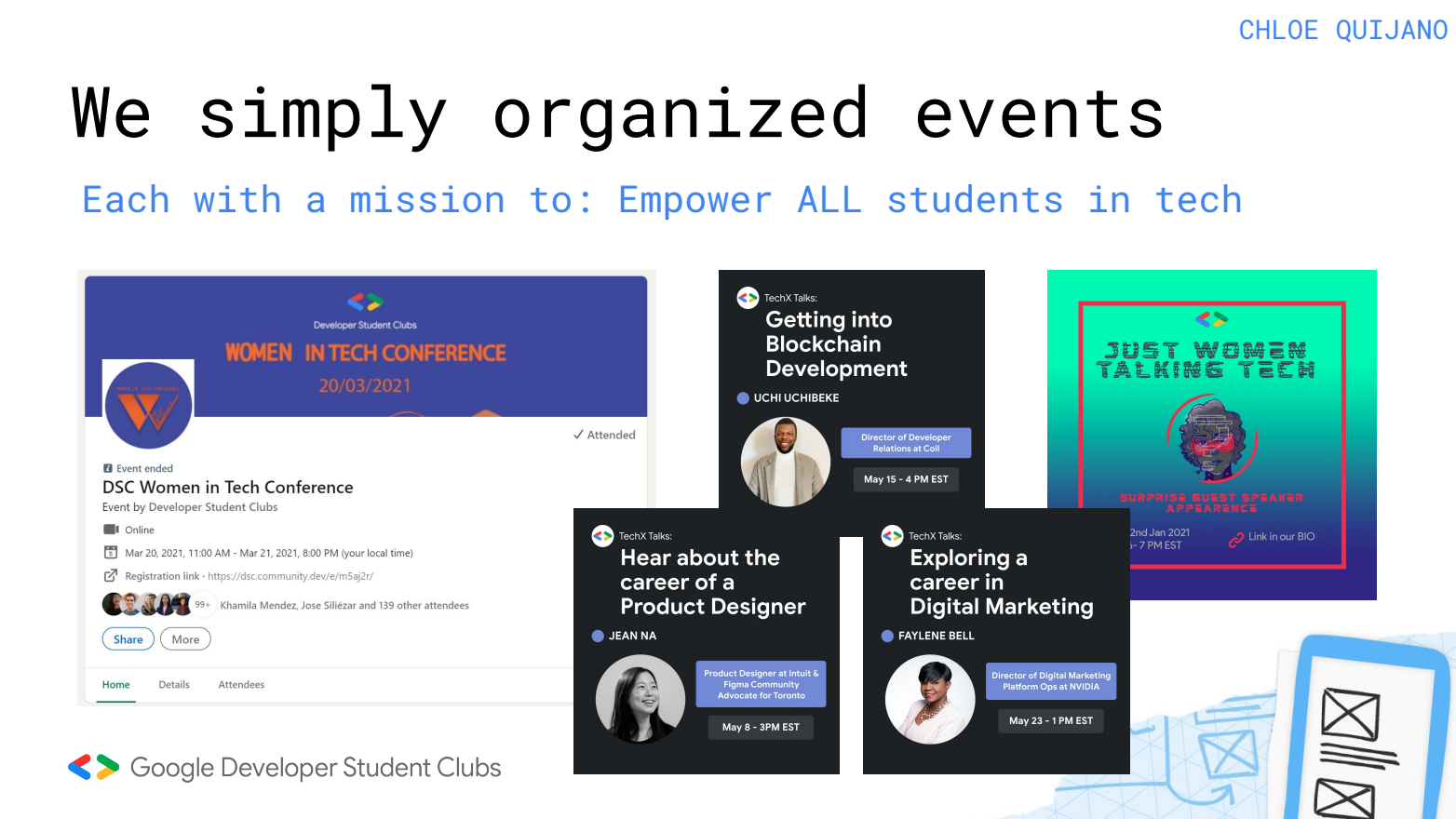
Examples of recent GDSC events at the University of Toronto
Chloe understood that greater encouragement and support can have long-term effects—not only while students are in school, but throughout their careers. “Showing more representation in tech greatly increases our confidence, innovation, and performance,” she points out. “Especially in academic environments, having a sense of belonging and community with role models for students to look up to can lead to success in tech together.”
Looking Ahead: Growth, Inspiration, and Connections
Having completed her term as the GDSC lead for the University of Toronto earlier this year, Chloe says the experience and her continuing involvement with the group has been instrumental in making her the student developer she is today. “I've been able to connect with student leaders globally from diverse backgrounds, academically, professionally, and culturally. It's inspiring to work alongside students who have unique perspectives.”
Such connections have become especially meaningful as she takes steps toward her post-graduation career, such as her first internship last summer, where she worked closely with several women software engineers at Microsoft. “Getting to work with them was really exciting and motivating,” she says.
Chloe can already see how her GDSC experience will support her professional development while also inspiring others to join the field. “I’m always going to be looking to become a better leader,” she says. “And then maybe other students like me who are looking for someone who looks like them, they’ll see me and think, ‘Yes, it’s possible.’”
Ultimately, Chloe says, helping others brings its own rewards: “Success takes care of those who create success for others.”
If you’re a student and would like to join a Google Developer Student Club community, look for a chapter near you here.
Source: Google Developers Blog
Extend Google Apps Script with your API library to empower users
Posted by Keith Einstein, Product Manager
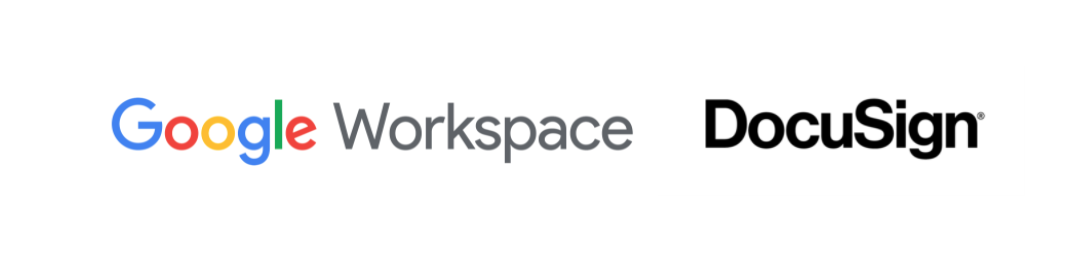
Google is proud to announce the availability of the DocuSign API library for Google Apps Script. This newly created library gives all Apps Script users access to the more than 400 endpoints DocuSign has to offer so they can build digital signatures into their custom solutions and workflows within Google Workspace.
The Google Workspace Ecosystem
Last week at Google Cloud Next ‘21, in the session “How Miro, DocuSign, Adobe and Atlassian are helping organizations centralize their work”, we showcased a few partner integrations called add-ons, found on Google Workspace Marketplace. The Google Workspace Marketplace helps developers connect with the more than 3 billion people who use Google Workspace—with a stunning 4.8 billion apps installed to date. That incredible demand is fueling innovation in the ecosystem, and we now have more than 5,300 public apps available in the Google Workspace Marketplace, plus thousands more private apps that customers have built for themselves. As a developer, one of the benefits of an add-on is that it allows you to surface your application in a user-friendly manner that helps people reclaim their time, work more efficiently, and adds another touchpoint for them to engage with your product. While building an add-on enables users to frictionlessly engage with your product from within Google Workspace, to truly unlock limitless potential innovative companies like DocuSign are beginning to empower users to build the unique solutions they need by providing them with a Google Apps Script Library.
Apps Script enables Google Workspace customization
Many users are currently unlocking the power of Google Apps Script by creating the solutions and automations they need to help them reclaim precious time. Publishing a Google Apps Script Library is another great opportunity to bring a product into Google Workspace and gain access to those creators. It gives your users more choices in how they integrate your product into Google Workspace, which in turn empowers them with the flexibility to solve more business challenges with your product’s unique value.
Apps Script libraries can make the development and maintenance of a script more convenient by enabling users to take advantage of pre-built functionality and focus on the aspects that unlock unique value. This allows innovative companies to make available a variety of functionality that Apps Script users can use to create custom solutions and workflows with the features not found in an off-the-shelf app integration like a Google Workspace Add-on or Google Chat application.
The DocuSign API Library for Apps Script
One of the partners we showcased at Google Cloud Next ‘21 was DocuSign. The DocuSign eSignature for Google Workspace add-on has been installed almost two-million times. The add-on allows you to collect signatures or sign agreements from inside Gmail, Google Drive or Google Docs. While collecting signatures and signing agreements are some of the most common areas in which a user would use DocuSign eSignature inside Google Workspace, there are many more features to DocuSign’s eSignature product. In fact, their eSignature API has over 400 endpoints. Being able to go beyond those top features normally found in an add-on and into the rest of the functionality of DocuSign eSignature is where an Apps Script Library can be leveraged.
And that’s exactly what we’re partnering to do. Recently, DocuSign’s Lead API Product Manager, Jeremy Glassenberg (a Google Developer Expert for Google Workspace) joined us on the Totally Unscripted podcast to talk about DocuSign’s path to creating an Apps Script Library. At the DocuSign Developer Conference, on October 27th, Jeremy will be teaming up with Christian Schalk from our Google Cloud Developer Relations team to launch the DocuSign Apps Script Library and showcase how it can be used.
With the DocuSign Apps Script Library, users around the world who lean on Apps Script to build their workplace automations can create customized DocuSign eSignature processes. Leveraging the Apps Script Library in addition to the DocuSign add-on empowers companies who use both DocuSign and Google Workspace to have a more seamless workflow, increasing efficiency and productivity. The add-on allows customers to integrate the solution instantly into their Google apps, and solve for the most common use cases. The Apps Script Library allows users to go deep and solve for the specialized use cases where a single team (or knowledge worker) may need to tap into a less commonly used feature to create a unique solution.
See us at the DocuSign Developer Conference
The DocuSign Apps Script Library is now available in beta and if you’d like to know more about it drop a message to [email protected]. And be sure to register for the session on "Building a DocuSign Apps Script Library with Google Cloud", Oct 27th @ 10:00 AM. For updates and news like this about the Google Workspace platform, please subscribe to our developer newsletter.
Source: Google Developers Blog
Google Smart Home Developer Summit – New tools and features to build, innovate, and grow with Google
Posted by Michele Turner, Senior Director of Product for Google’s Smart Home Ecosystem
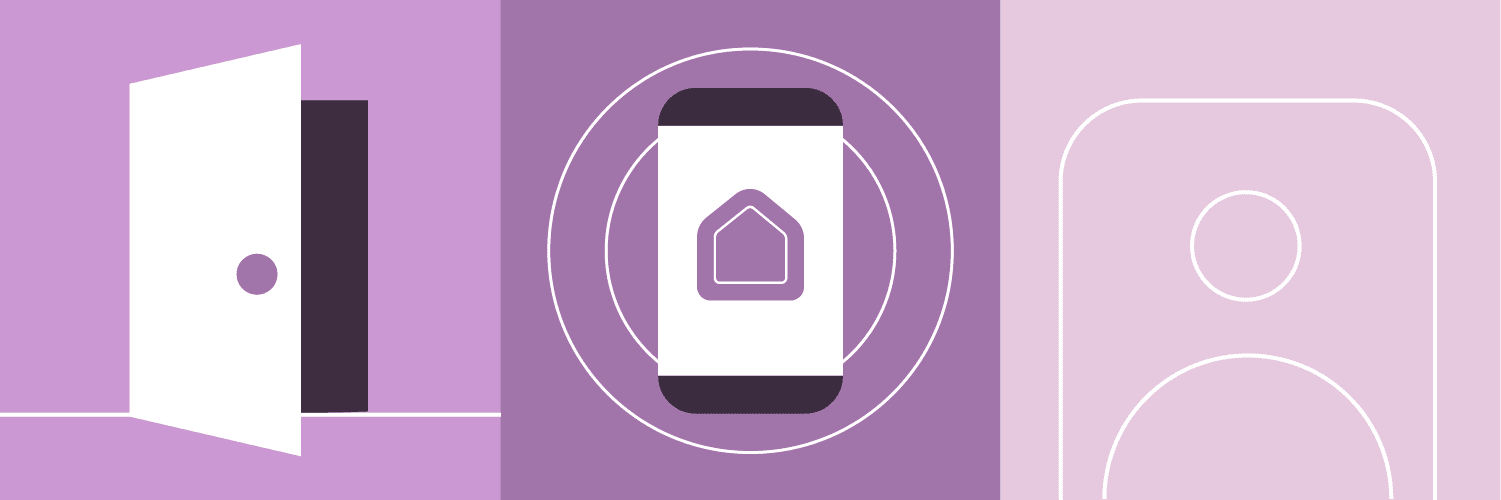
Earlier this year at Google I/O, we told you that our goal is to make Google the best place for smart home developers like you to build, innovate, and grow. Today, I'm excited to show you all the new ways we're improving the tools and opportunities you'll have to build you best experiences with Google, by:
- Expanding our platform and tools to make it easier for you to learn and build devices that do more with Google.
- Providing a site where you can preview the new tools that are coming over the next year to help you build your devices, apps, and integrations.
- Supporting Matter & Thread across our entire ecosystem, including Nest and Android.
- Developing more automation capabilities, including the ability to build suggested routines for your users.
- Helping you differentiate with Google and connect to more users.
(re) Introducing “Google Home”
Our journey as an ecosystem started five years ago with the Google Home speaker and Google Assistant. It has grown into a powerful platform, with support for new smart speakers and displays, Android, Nest, and the Google Home app. It also includes an ecosystem of tens of thousands of devices made by partners and developers like you, enabling Google users to engage with over 200 million devices, and making the smart home more than the sum of its parts.
We’re bringing all of this together, and announcing a new, but familiar name, for our entire smart home platform and developer program, that helps users and developers do more with Google – Google Home. By bringing our platform and tools under the same roof, it gives us a simpler way to show you why and how integrating your devices with Google Home makes them more accessible and helpful across the Google ecosystem.
New Google Home Developer Center
Launching early next year, you’ll have access to our new Google Home Developer Center that will have everything you need to learn and build smart home devices, applications, and automations with Google. It’s a total redesign of our developer site and console, focused on major upgrades to navigation, and new self-serve tools for both developers and their business teams.
The developer center will have tools for each step of development, deployment, and analytics, including:
- Building Matter devices
- Customizing setup of your devices in Android and the Google Home app
- Creating automations and routines
- Building Android apps with Matter
- Testing and certification
- New tools for analytics & performance monitoring
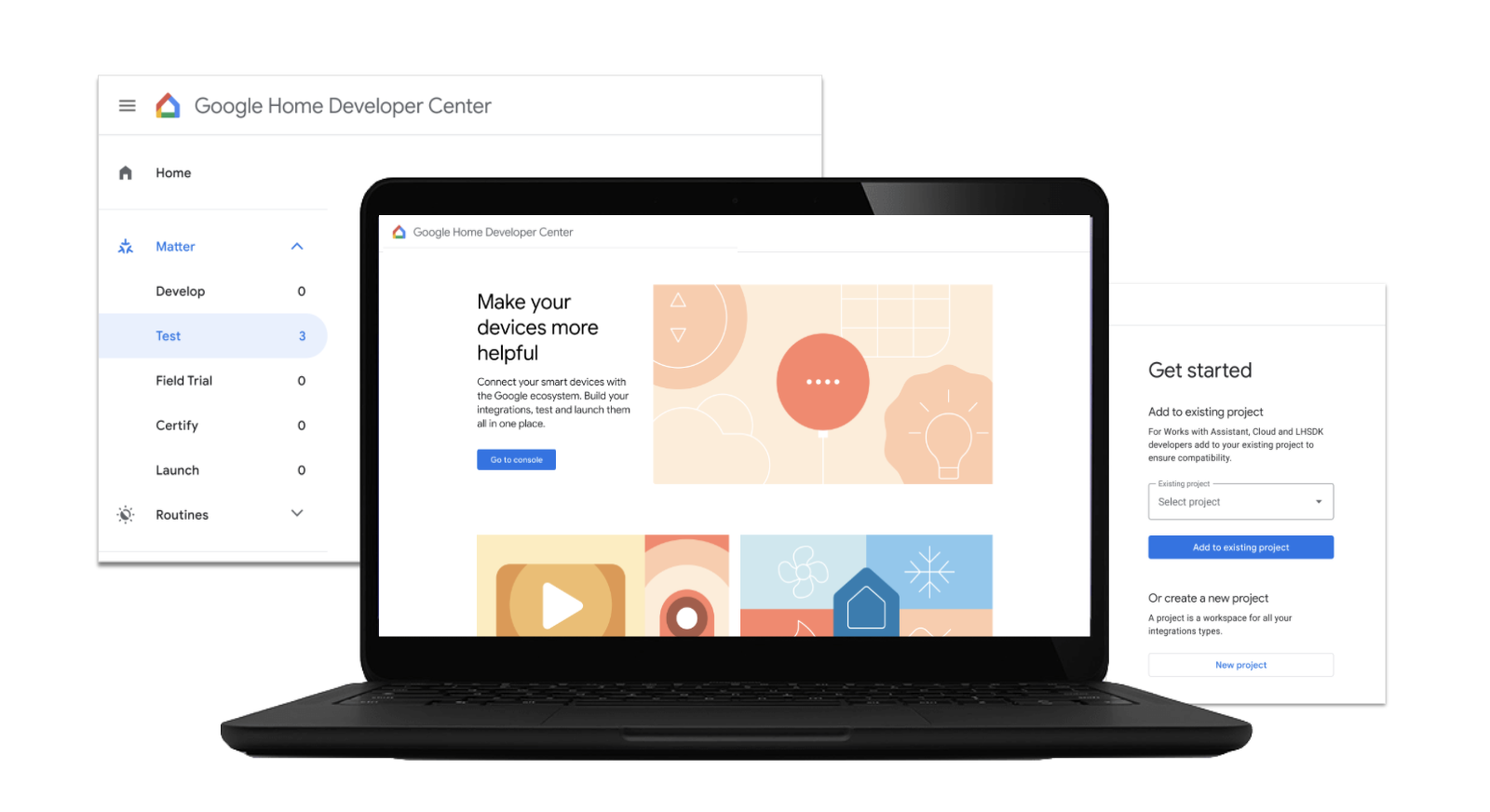
Quickly build and integrate with Matter
One of the most important new capabilities we’re bringing to our developers is the ability to quickly build and integrate Matter devices. We’re continuing to collaborate with other leading and innovative companies from across the industry to develop Matter — the new, universal, open smart home application protocol that makes it easy to build, buy, and set up smart home devices with any Matter ecosystem or app. We’re also adding Matter as a powerful new way to connect your devices to Google Home and your Android apps.
To make sure users are ready for your Matter devices, we’ll update Nest and Android devices with Matter support, following the launch of the new standards. That means when you build devices with Matter, they can be easily set up and controlled by millions of users everywhere they interact with Google, including Nest speakers and displays, the Google Assistant, and of course Android devices. To make sure you’re ready to build your best Matter-enabled experiences with Google, we’re adding support for Matter in the Google Home Developer Center, and rolling out new tools for Matter development across Google Home and Android, including two new SDKs.
New Google Home Device SDK for Matter devices
The first is the Google Home Device SDK — the fastest way to develop Matter devices, enabling seamless setup, control, and interoperability.
The open source Matter specification and SDK will ensure everyone is starting from the same code base. But building innovative, quality experiences goes beyond sharing the same connectivity protocol. The Google Home Device SDK complements the open-source libraries and simplifies building Matter devices to work seamlessly with Google, including configuring your device with Assistant, improving quality with logging, and adding tools to interact and test with Google devices. This helps you build a more responsive, reliable end-to-end experience for users. We’ll also be adding new capabilities that allow you to innovate with the SDK.
To make your development even easier, we’re also delivering the Google Home IDE to build your smart home devices and connect them to Google in a familiar way. For developers using Visual Studio Code to develop smart home devices, you can easily leverage our tools in that environment by installing the new Google Home IDE, which complements your existing extensions and tools in this popular editor.
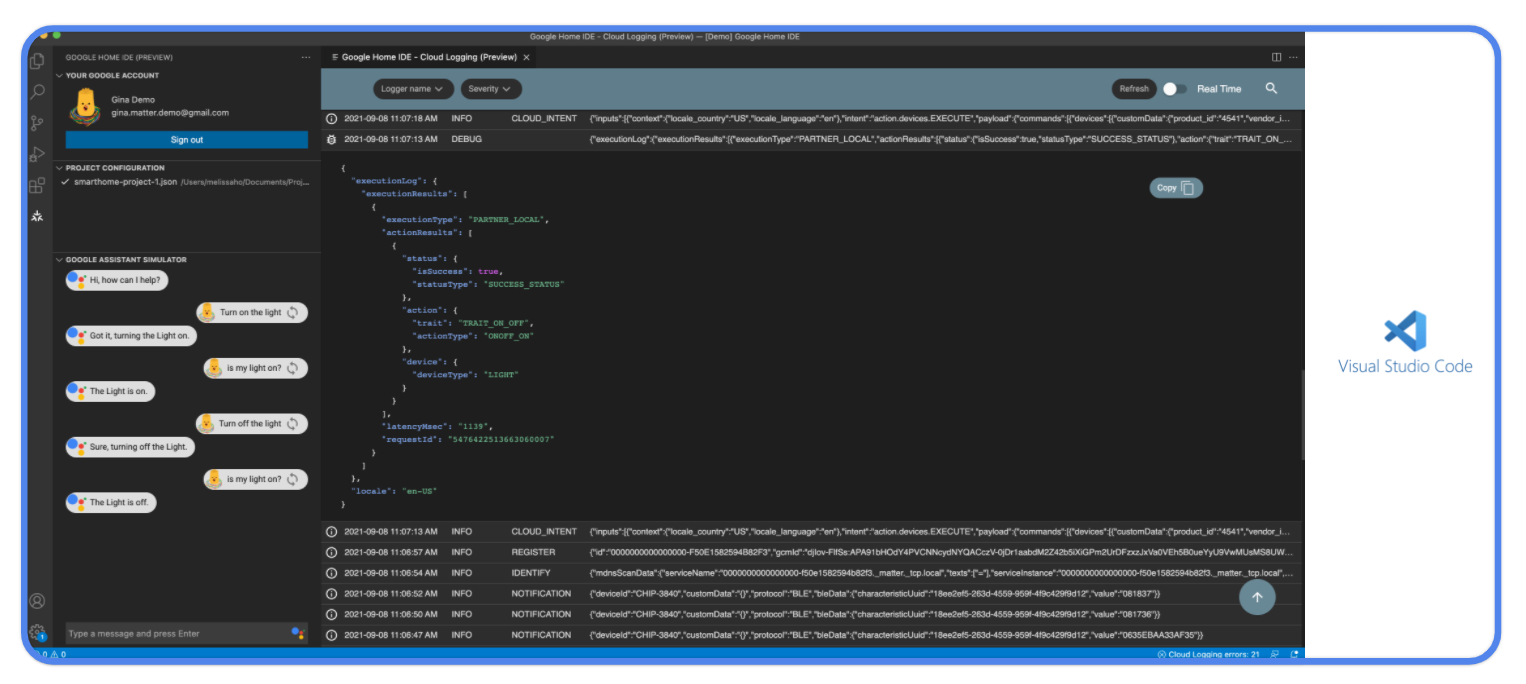
Native Android Support via Google Play Services and a new Google Home Mobile SDK
Mobile devices are an important smart home tool for users, and are critical to how users set up, manage, and control the devices in their home. To make app experiences more seamless, and help your users experience the magic of your device as quickly as possible, we’re building Matter directly into Android, and announcing support for Matter through Google Play services.
One of the key benefits this enables is seamless Matter device setup flows in Android, letting users connect new Matter devices over WiFi and Thread as easily as a new pair of headphones. You’ll be able to customize that setup flow with branding and device descriptions. With just a few taps, users will be able to link your devices to your app, the Google Home app, and other Matter apps they’ve installed. Of course, when users connect your device to Google, it automatically shows up in the Google Home app, on Android controls for smart home devices, and is controllable with the Google Assistant on Android, without additional development.
We’re also creating new tools to accelerate your development with the Google Play services Matter APIs, using the new Google Home Mobile SDK. Building a Matter-native app on Android lets users link their smart home devices to your app during the setup process, or later in their journey, with a few easy taps - with no need for account linking.
We’re already well underway building Matter integrations with many of the leaders in the smart home industry, and helping their Matter devices do more with Google, with many more to follow.
Inspire engagement with Suggested Routines
Whether via Matter or existing integration paths, being able to easily and reliably connect your devices to Google helps users build their smart homes. For developers, automations allow users to do more with your devices.
We want to help you easily combine them with other devices into coordinated routines, and to use context and triggers to increase their usefulness and engagement with the help of Google’s intelligence. So in our new Developer Center, we’ll enable you to create your own suggested routines that users can easily discover directly in the Google Home app. Your routines can carry your brand, suggest new ways for users to engage with your devices, and enhance them by coordinating them with other devices and context signals in the home.
Do more with Google Home
This is just the start of new ways we’re enabling your devices and brands to do more with Google Home. We know that for device makers, compatibility with Google Home is an important way to engage your users. But you want to make sure that your brand, products, and innovations are front and center with your users, to help them get the most from the experiences you’ve built.
That’s why all of the new tools we’re building help you to go beyond just compatibility with Google Home — and empower you to build your best, most engaging experiences with Google.
- Customizable setup flows built into Android and Google Home that let your users experience the magic of your device with just a few taps right out of the box.
- Native Matter apps on Android your users can discover and connect to in one streamlined setup flow.
- Suggested routines to help your users do more with your devices.
- New ways for users to discover and use your devices’ capabilities within the Google Home app.
- the new Google Home Developer Center that brings developer and marketing tools together in one place, to help you and your team quickly bring all this to market.
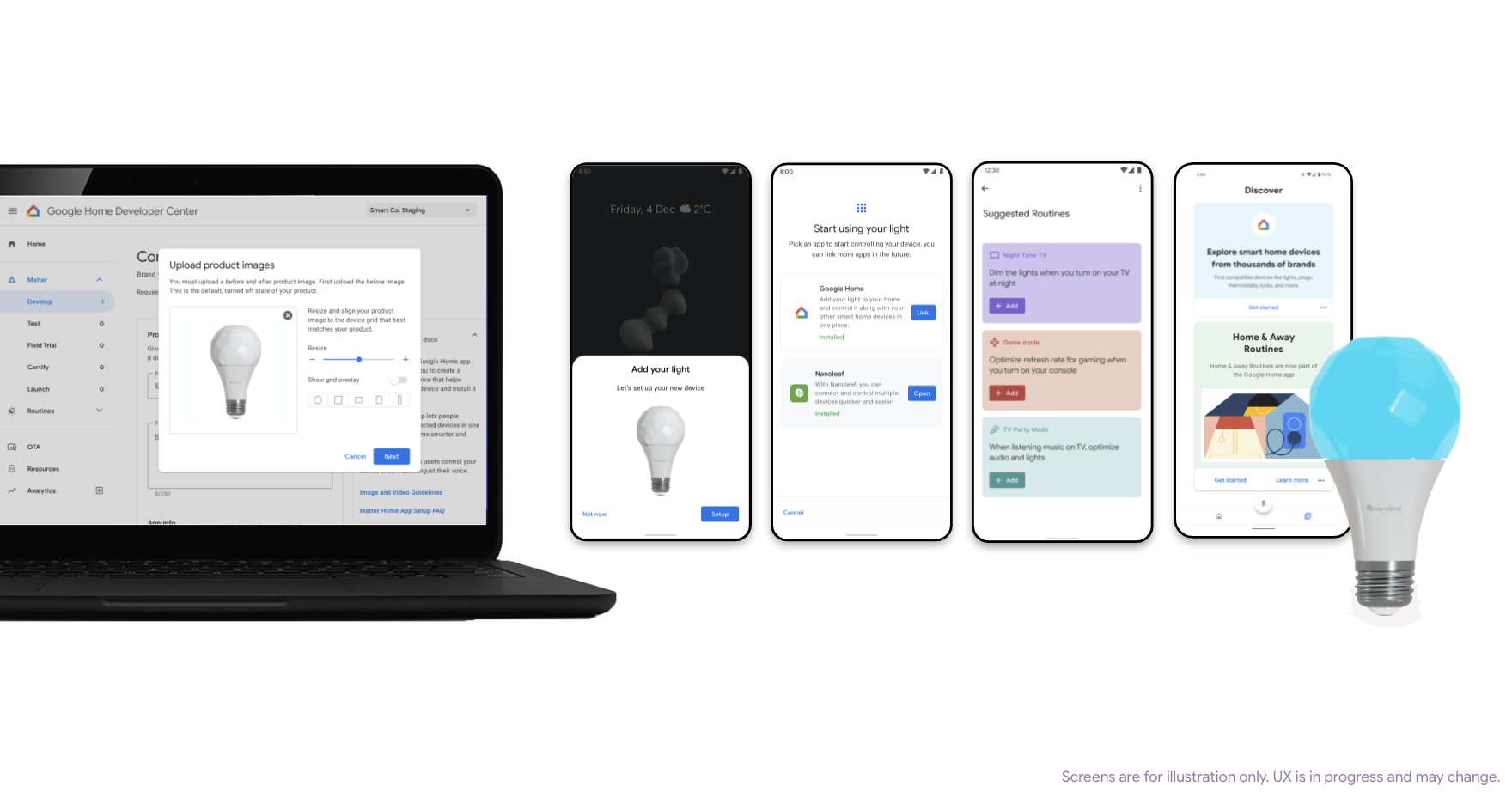
Support user growth and discovery
Of course, when you’ve built those great experiences, you want to tell everyone about them! For users that haven’t discovered your devices yet, we’re leveraging the power of Google to help users learn about your devices, and bring them home.
Earlier this year, we launched our new smart home directory on web and mobile that has seen great user engagement. This new site gives consumers an easy to use resource for discovering smart devices compatible with Google, and the experiences they can create with them, whether with a single device or using multiple devices together with automations and routines. We’re continuing to expand the site with more use cases, addressing the needs of both beginners and more sophisticated users looking to grow their smart homes and get more out of them.
We’ll have more to share with you over the coming months! Visit developers.googoe.com/home to read more about our announcements today and sign up for updates. We can’t wait to see what you build!
Source: Google Developers Blog
Migrating App Engine push queues to Cloud Tasks
Posted by Wesley Chun (@wescpy), Developer Advocate, Google Cloud
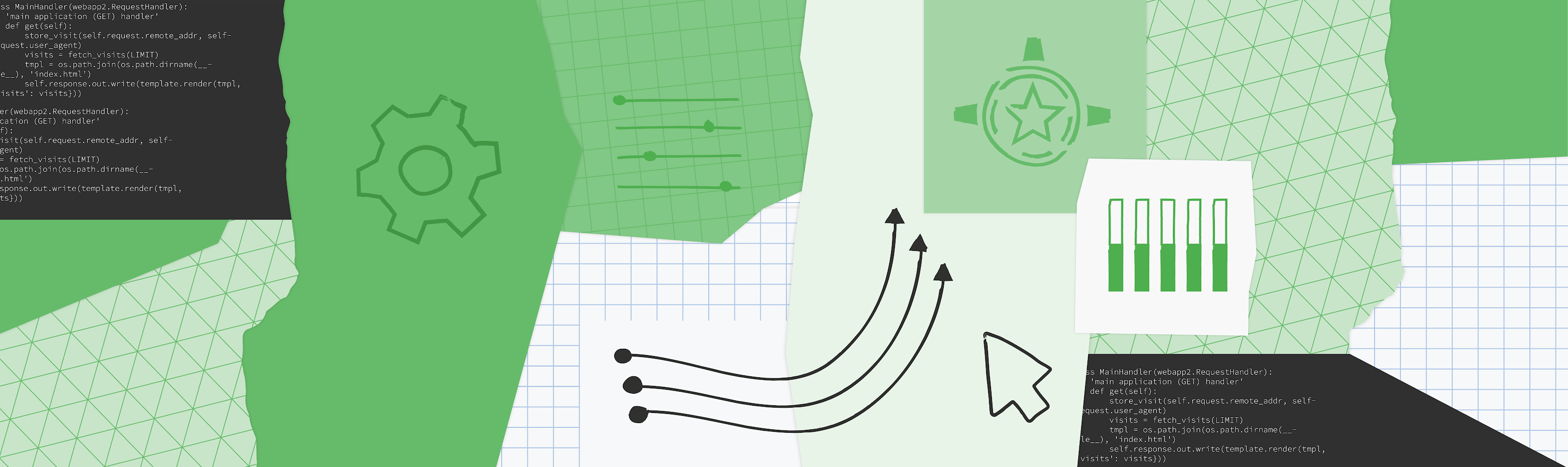
Introduction
The previous Module 7 episode of Serverless Migration Station gave developers an idea of how App Engine push tasks work and how to implement their use in an existing App Engine ndb Flask app. In this Module 8 episode, we migrate this app from the App Engine Datastore (ndb
) and Task Queue (taskqueue
) APIs to Cloud NDB and Cloud Tasks. This makes your app more portable and provides a smoother transition from Python 2 to 3. The same principle applies to upgrading other legacy App Engine apps from Java 8 to 11, PHP 5 to 7, and up to Go 1.12 or newer.
Over the years, many of the original App Engine services such as Datastore, Memcache, and Blobstore, have matured to become their own standalone products, for example, Cloud Datastore, Cloud Memorystore, and Cloud Storage, respectively. The same is true for App Engine Task Queues, whose functionality has been split out to Cloud Tasks (push queues) and Cloud Pub/Sub (pull queues), now accessible to developers and applications outside of App Engine.
Migrating App Engine push queues to Cloud Tasks video
Migrating to Cloud NDB and Cloud Tasks
The key updates being made to the application:
- Add support for Google Cloud client libraries in the app's configuration
- Switch from App Engine APIs to their standalone Cloud equivalents
- Make required library adjustments, e.g., add use of Cloud NDB context manager
- Complete additional setup for Cloud Tasks
- Make minor updates to the task handler itself
The bulk of the updates are in #3 and #4 above, and those are reflected in the following "diff"s for the main application file:
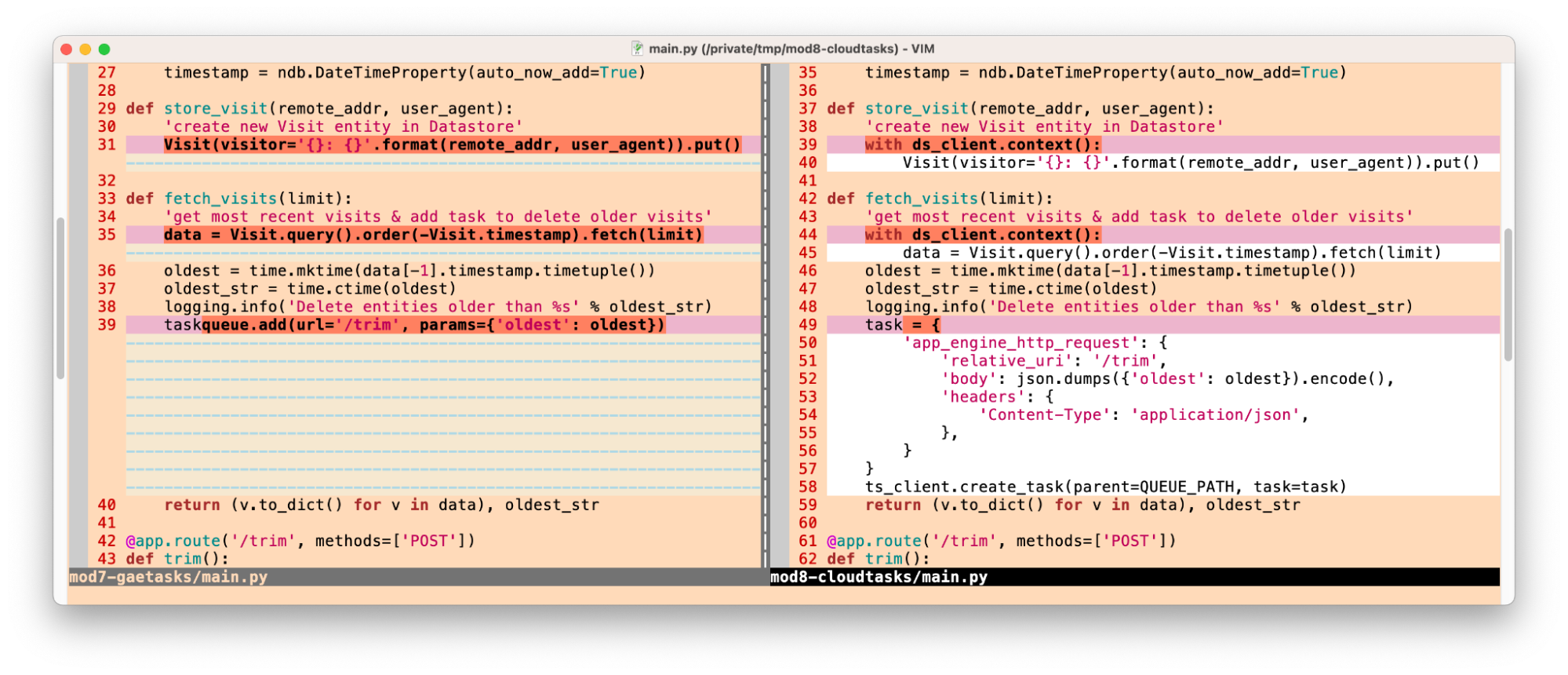
Primary differences switching to Cloud NDB & Cloud Tasks
With these changes implemented, the web app works identically to that of the Module 7 sample, but both the database and task queue functionality have been completely swapped to using the standalone/unbundled Cloud NDB and Cloud Tasks libraries… congratulations!
Next steps
To do this exercise yourself, check out our corresponding codelab which leads you step-by-step through the process. You can use this in addition to the video, which can provide guidance. You can also review the push tasks migration guide for more information. Arriving at a fully-functioning Module 8 app featuring Cloud Tasks sets the stage for a larger migration ahead in Module 9. We've accomplished the most important step here, that is, getting off of the original App Engine legacy bundled services/APIs. The Module 9 migration from Python 2 to 3 and Cloud NDB to Cloud Firestore, plus the upgrade to the latest version of the Cloud Tasks client library are all fairly optional, but they represent a good opportunity to perform a medium-sized migration.
All migration modules, their videos (when available), codelab tutorials, and source code, can be found in the migration repo. While the content focuses initially on Python users, we will cover other legacy runtimes soon so stay tuned.
Source: Google Developers Blog
Announcing DevFest 2021
Posted by Erica Hanson, Global Program Manager for Google Developer Communities
DevFest season has officially started! From now through the end of the year, developers from all around the world are coming together for DevFest 2021, the biggest global event for developers, focusing on community-led learning on Google technologies. Hosted by Google Developer Groups (GDG) all across the globe, DevFest events are uniquely curated by their local GDG organizers to fit the needs and interests of the local community.
The mission
This year, DevFest 2021 inspires local developers to learn and connect as a community by exploring how to use Google technology to accelerate economic impact. In light of COVID-19, the global economy has shrunk and millions of jobs have been lost. Developers are the backbone of technology, and they play a pivotal role in the recovery of the global economy. In fact, expanding the impact of developers has never been more important!
Luckily, DevFest is the perfect opportunity for Google Developer Groups to show up for developers and their communities during such a challenging time. At DevFest 2021, GDGs and attendees will have the opportunity to explore how to use technology for good where it’s needed most.
Accelerating local economic recovery looks different across the globe, and GDGs hosting DevFest events are encouraged to consider the challenges their specific regions may be facing. For example, GDGs may choose to focus their DevFest events on building solutions that help local businesses grow, or they may prioritize upskilling their community by sharing technical content to help developers become industry ready. Whether it be through technical talks delivered in local languages or by simply meeting fellow local developers, DevFest 2021 will leave attendees feeling empowered to drive positive change in their communities.
What to expect
One of DevFest’s greatest strengths remains the passionate speakers who participate in DevFest events all across the globe. These speakers, often developers themselves, come from various backgrounds, perspectives, and skill levels to create a rich and rewarding experience for attendees. DevFest sessions are hosted in local languages in many different parts of the world.
This DevFest season, attendees will receive career support and mentorship opportunities from senior developers, including speakers from Google, Google Developer Group leaders, Google Developer Experts, and Women Techmakers.
Hands-on demos, workshops, and codelabs will cover a wide variety of technologies, including Android, Google Cloud Platform, Machine Learning with TensorFlow, Web.dev, Firebase, Google Assistant, and Flutter. Through these events, developers will learn how Google technologies help them build solutions that make a difference.
Google Developers is proud to support the community-led efforts of Google Developer Groups during this flagship annual event. DevFest is powered by a global network of passionate GDG community organizers who volunteer their time and efforts to help developers grow together, and this event wouldn’t be possible without them.
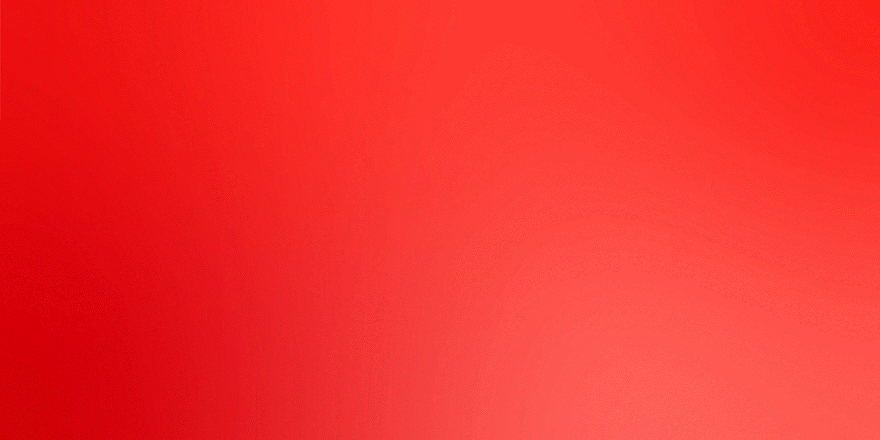
Coming together
During DevFest 2020, 125,000+ developers participated across 700+ DevFests in 100+ countries. DevFest 2021 is already in full swing, with thousands of attendees across the globe collaborating with like-minded developers, learning new technologies, and building solutions to uplift their communities. Whether you’re looking to explore the latest Google technologies, level up your career, or innovate for impact, there is a DevFest event for you.
Find a DevFest near you here, and use #DevFest to join the conversation on social media.
Source: Google Developers Blog
“Bowling” automatic disapproved ads remover
Posted by Elad Ben-David, Nir Kalush, Dvir Kalev, Chen Yogev, Tzahi Zilberstein, Eliran Drucker
Tagline:
In light of the new policy that might cause accounts suspension, Bowling is a mitigation tool allowing clients to act and remove disapproved ads before risking account suspension.
The tool audits (and offers the option to delete) disapproved ads that may lead eventually to account suspension in perpetuity.
Business Challenge:
Starting Oct 2021 Google is introducing a new strike-based system to enforce against advertisers who repeatedly violate Google Ads policies (read more about the change here).
An advertiser’s first policy violation will result in a warning. If we detect continued violation of our policies advertisers will receive a notice they got a strike on the account, with a maximum of three strikes. The penalties applied with each strike will progressively rise. Temporary account holds will be applied for the first and second strikes, while the third strike will cause an account suspension.
Advertisers with hundreds of accounts and billions of search keywords lack the bandwidth to monitor each violation, thus might receive repeated strikes and get suspended.
Solution Overview:
“3 Strike Bowling” is an automated solution which identifies and gathers all relevant disapproved apps and includes the option to remove violating ads, in order to ensure compliance and avoid account suspension.
*The user can define an exclusion list of policy topics to ignore and not remove.
It’s a simple Python script, which can be run in either of the following modes:
- “Audit Mode”- export all the disapproved ads without deleting them;
- “Remove Mode” - delete all the disapproved ads and log the disapproved ads’ details.
There are a few output files (see here) which are saved locally under the “output” folder and optionally on BigQuery as well ( “google_3_strikes” dataset).
Skills Required:
- Install technical prerequisites.
- In high level: Python 3.9+, Google Ads API, OAuth 2.0
- Run one command line via the terminal.
Google Products Used:
- Google Ads API
- BigQuery API (optional)
Estimated time to implement the solution: ~2h
Implementation instructions: View on github