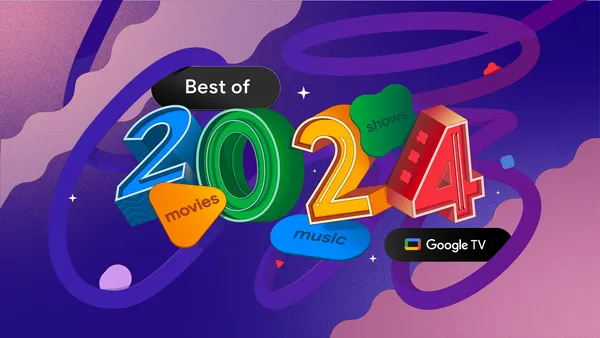
2024’s greatest hits on Google TV
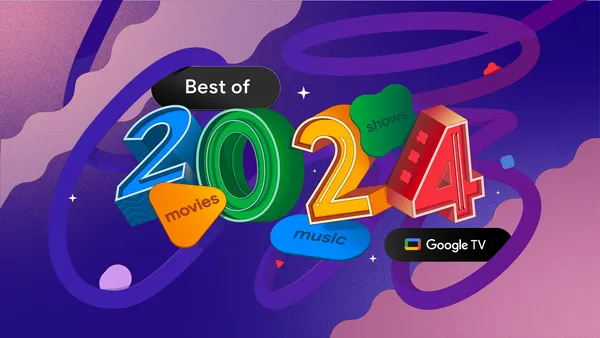
Posted by Neelansh Sahai - Developer Relations Engineer
Did you know that, on average, 40% of the people in the US reset or replace their smartphones every year? This frequent device turnover presents a challenge – and an opportunity – for maintaining strong user relationships. When users get a new phone, the friction of re-entering login credentials can lead to frustration, app abandonment, and churn.
To address this issue, we're introducing Restore Credentials, a new feature of Android’s Credential Manager API. With Restore Credentials, apps can seamlessly onboard users to their accounts on a new device after they restore their apps and data from their previous device. This makes the transition to a new device effortless and fosters loyalty and long term relationships.
On top of all this, there's no developer effort required for the transfer of a restore key from one device to the other, as this process is tied together with the android system’s backup and restore mechanism. However, if you want to login your users silently as soon as the restore is completed, you might want to implement BackupAgent and add your logic in the onRestore callback. The experience is delightful - users will continue being signed in as they were on their previous device, and they will be able to get notifications to easily access their content without even needing to open the app on the new device.
Some of the benefits of the Restore Credentials feature include:
The Restore Credentials feature enables seamless user account restoration on a new device. This process occurs automatically in the background during device setup when a user restores apps and data from a previous device. By restoring app credentials, the feature allows the app to sign the user back in without requiring any additional interaction.
The credential type that’s supported for this feature is called restore key, which is a public key compatible with passkey / FIDO2 backends.
On the old device:
On the new device:
Note: You should delete the restore key as soon as the user signs out. You don’t want your user to get stuck in a cycle of signing out intentionally and then automatically getting logged back in.
Using the Jetpack Credential Manager let you create, get, and clear the relevant Restore Credentials:
Restore Credentials is available through the Credential Manager Jetpack library. The minimum version of the Jetpack Library is 1.5.0-beta01, and the minimum GMS version is 242200000. For more on this, refer to the Restore Credentials DAC page. To get started, follow these steps:
1. Add the Credential Manager dependency to your project.
// build.gradle.kts implementation("androidx.credentials:credentials:1.5.0-beta01")
2. Create a CreateRestoreCredentialRequest object.
// Fetch Registration JSON from server // Same as the registrationJson created at the time of creating a Passkey // See documentation for more info val registrationJson = ... // Create the CreateRestoreCredentialRequest object // Pass in the registrationJSON val createRequest = CreateRestoreCredentialRequest( registrationJson, /* isCloudBackupEnabled = */ true )
NOTE: Set the isCloudBackupEnabled flag to false if you want the restoreKey to be stored locally and not in the cloud. It’s set as true by default
3. Call the createCredential() method on the CredentialManager object.
val credentialManager = CredentialManager.create(context) // On a successful authentication create a Restore Key // Pass in the context and CreateRestoreCredentialRequest object val response = credentialManager.createCredential( context, createRestoreRequest )
4. When the user sets up a new device, call the getCredential() method on the CredentialManager object.
// Fetch the Authentication JSON from server val authenticationJson = ... // Create the GetRestoreCredentialRequest object val options = GetRestoreCredentialOption(authenticationJson) val getRequest = GetCredentialRequest(Immutablelist.of(options)) // The restore key can be fetched in two scenarios to // 1. On the first launch of app on the device, fetch the Restore Key // 2. In the onRestore callback (if the app implements the Backup Agent) val response = credentialManager.getCredential(context, getRequest)
If you're using a backup agent, perform the getCredential part within the onRestore callback. This ensures that the app's credentials are restored immediately after the app data is restored.
5. When the user signs out of your app, call the clearCredentialState() method on the CredentialManager object.
// Create a ClearCredentialStateRequest object val clearRequest = ClearCredentialStateRequest(/* requestType = */ 1) // On user log-out, clear the restore key val response = credentialManager.clearCredentialState(clearRequest)
The Restore Credentials feature provides significant benefits, ensuring users experience a smooth transition between devices, and allowing them to log in quickly and easily through backup agents or restore key checks. For developers, the feature is straightforward to integrate and leverages existing passkey server-side infrastructure. Overall, Restore Credentials is a valuable tool that delivers a practical and user-friendly authentication solution.
This blog post is a part of our series: Spotlight Week: Passkeys. We're providing you with a wealth of resources through the week. Think informative blog posts, engaging videos, practical sample code, and more—all carefully designed to help you leverage the latest advancements in seamless sign-up and sign-in experiences.
With these cutting-edge solutions, you can enhance security, reduce friction for your users, and stay ahead of the curve in the rapidly evolving landscape of digital identity. To get a complete overview of what Spotlight Week has to offer and how it can benefit you, be sure to read our overview blog post.
Earlier this year #WeArePlay went on a virtual tour of Australia and the U.S. to spotlight the stories of app and game founders. Today, we’re rounding up our tour across the world with the last stop for 2024: Japan. From an app that uses AI to promote early health intervention to one that’s turning litter picking into a social movement, meet the 49 apps and games founders building growing businesses on Google Play.
Let’s take a quick road trip across Japan by reading some of my favorite stories.
When Fujio was a child, his love for environmental books ignited his passion for protecting nature. Later, while traveling through different countries, he was shocked to find litter everywhere, even in remote jungles. This experience inspired him to create Pirika – named after the Ainu word for “beautiful” – a social platform that encourages people to pick up litter, document it with photos, and geotag locations to track problem areas. With over over 360 million pieces of trash collected globally, Pirika is fostering a nationwide movement towards cleaner communities. Fujio plans on expanding the app’s reach worldwide, mobilizing communities to fight pollution collectively.
Self-taught game developer and illustrator CHARROOM turned her passion for mini-games into a full-time career. Her latest project, Sushi Food Cart, combines two of her favorite things: sushi and cats. In this fun cooking game, players manage a sushi food cart, preparing and serving sushi to customers quickly and accurately. The game features quirky cat characters, time-management challenges, and colorful art. As players progress, they unlock new recipes, upgrades, and meet new cat characters. For Char, creating apps is her ‘ikigai’—her purpose in life. She’s currently working on a new restaurant game to continue spreading her playful approach to gaming.
When entrepreneur Kota decided to create a healthcare app, he partnered with his high school friend and roommate, Dr. Yoshinori. Combining Kota’s tech expertise with Yoshinori’s medical knowledge, they developed Ubie, an AI-powered symptom checker that allows people to research their symptoms and connect with medical specialists. The app promotes early detection and intervention by offering them tailored information on potential health concerns and guidance on finding care. Ubie's goal is not just limited to symptom checking; the founders plan to expand its services to include tracking treatments and managing day-to-day healthcare needs alongside transforming Ubie into a global brand.
Takuji has always been passionate about travel and music, but when he learned programming 15 years ago, he discovered a new creative outlet. Together with his brother, Shohei, he launched IzumiArtisan from their parents' house in Osaka. The duo specializes in creating escape room games, heavily influenced by the detective stories Takuji loves. Their popular game, Rime, is filled with mysterious puzzles and plot twists and has been translated into 18 languages, capturing a global audience. The brothers are now working on a new title inspired by the American Underground Railroad.
Discover more #WeArePlay stories from Japan, and stories from across the globe.
Android 16 Developer Preview 1 is available now to test with your apps. This is the start of Android having more frequent API releases as part of our effort to drive faster innovation in apps and devices.
In addition to new developer APIs, the Q4 minor release will pick up feature updates, optimizations, and bug fixes; it will not include any app-impacting behavior changes.
We'll continue to have quarterly Android releases. The Q1 and Q3 updates in-between the API releases will provide incremental updates to help ensure continuous quality. We’re actively working with our device partners to bring the Q2 release to as many devices as possible.
Guarding a code block with a check for Android's API level is done today using the SDK_INT constant with VERSION_CODES. This will continue to be supported for major Android releases.
if (SDK_INT >= VERSION_CODES.BAKLAVA) { // Use APIs introduced in Android 16 }
The new SDK_INT_FULL constant can be used for API checks against both major and minor versions with the new VERSION_CODES_FULL enumeration.
if (SDK_INT_FULL >= VERSION_CODES_FULL.[MAJOR or MINOR RELEASE]) { // Use APIs introduced in a major or minor release }
You can also use the Build.getMinorSdkVersion() method to get just the minor SDK version.
val minorSdkVersion = Build.getMinorSdkVersion(VERSION_CODES_FULL.BAKLAVA)
These APIs have not yet been finalized and are subject to change, so please send us feedback if you have any concerns.
Note that there’s no change to the target API level requirements and the associated dates for apps in Google Play; our plans are for one annual requirement each year, and that will be tied to the major API level.
The photo picker provides a safe, built-in way for users to grant your app access to selected images and videos from both local and cloud storage, instead of their entire media library. Using a combination of Modular System Components through Google System Updates and Google Play services, it's supported back to Android 4.4 (API level 19). Integration requires just a few lines of code with the associated Android Jetpack library.
The developer preview includes new APIs that enable apps to embed the photo picker into their view hierarchy. This allows it to feel like a more integrated part of the app while still leveraging the process isolation that allows users to select media without the app needing overly-broad permissions. To maximize compatibility across platform versions and simplify your integration, you'll want to use the forthcoming Android Jetpack library if you wish to integrate the embedded photo picker.
The developer preview of Health Connect contains an early version of APIs supporting health records. This allows apps to read and write medical records in FHIR format with explicit user consent. This API is currently in an early access program. Sign up to be part of our early access program.
Android 16 incorporates the latest version of the Privacy Sandbox on Android, part of our ongoing work to develop technologies where users know their privacy is protected. Our website has more about the Privacy Sandbox on Android developer beta program to help you get started. Check out the SDK Runtime which allows SDKs to run in a dedicated runtime environment separate from the app they are serving, providing stronger safeguards around user data collection and sharing.
In addition to performing compatibility testing on the next major release, make sure that you're compiling your apps against the new SDK, and use the compatibility framework to enable targetSdkVersion-gated behavior changes as they become available for early testing.
The Android 16 Preview program runs from November 2024 until the final public release next year. At key development milestones, we'll deliver updates for your development and testing environments. Each update includes SDK tools, system images, emulators, API reference, and API diffs. We'll highlight critical APIs as they are ready to test in the preview program in blogs and on the Android 16 developer website.
We’re targeting Late Q1 of 2025 for our Platform Stability milestone. At this milestone, we’ll deliver final SDK/NDK APIs and also final internal APIs and app-facing system behaviors. We’re expecting to reach Platform Stability in March 2025, and from that time you’ll have several months before the official release to do your final testing. Visit our Android Developers site for details on the release timeline.
You can get started today with Developer Preview 1 by flashing a system image and updating the tools. We're looking for your feedback so please report issues and submit feature requests on the feedback page. The earlier we get your feedback, the more we can include in the final release.
For the best development experience with Android 16, we recommend that you use the latest preview of the Android Studio Ladybug feature drop. Once you’re set up, here are some of the things you should do:
We’ll update the preview system images and SDK regularly throughout the Android 16 release cycle. This initial preview release is for developers only and not intended for daily or consumer use, so we're making it available by manual download only. Once you’ve manually installed a preview build, you’ll automatically get future updates over-the-air for all later previews and Betas. Visit the Android developer website for further information on Android updates.
If you've already installed Beta 1 from the Android 15 QPR2 Beta program, you will not be able to move to the Android 16 Developer Preview program without wiping your device. Consider avoiding installing future betas to transition to the next developer preview build without a data wipe.
As we reach our Beta releases, we'll be inviting consumers to try Android 16 as well, and we'll open up enrollment for Android 16 in the Android Beta program at that time.
For complete information, visit the Android 16 developer site.
We're kicking off Spotlight Week with a deep dive into passkeys! This week we're partnering with the Chrome team to feature exciting announcements, insightful resources, and expert guidance on how to build seamless and secure authentication experiences for your apps.
Throughout Spotlight Week: Passkeys, we'll share content to help you understand and implement passkeys effectively. Expect technical deep dives, best practices for user experience, case studies from successful implementations, and answers to your questions.
We'll start off the week with resources to help you begin passkey integration. Check out these resources to get started!
Key resources include a quick video on passkey basics, updated UX guidelines for Credential Manager and passkeys, and an in-depth server-side implementation guide. We will introduce you to the Identity hub, a comprehensive resource for passkeys, passwords, Sign in with Google, authorization, and much more.
We'll share updated passkeys developer guidance, including migration guides, a new troubleshooting guide, and more. Highlights include a guide on migrating from legacy APIs to Credential Manager, technical details on FIDO2 attestation format changes, and a troubleshooting guide for common Credential Manager errors.
Developers can also share feedback through a passkeys survey to influence future improvements.
We'll go over some of the new Credential Manager capabilities, including improvements to autofill, single-tap sign-in, and the new Restore Credentials feature. Key updates include showing Credential Manager results as autofill suggestions, single-tap sign-in, Signal API for Chrome desktop and a Restore Credentials feature, which allows users to conveniently recover their saved login information in case of device loss or upgrades, ensuring uninterrupted access to their accounts. With Android 15, these additions streamline user authentication and reinforce security, making it easier for users to manage and access their credentials securely.
On Thursday at 9AM PT Spotlight Week: Passkeys will feature an #AskAndroid session to address your most pressing passkey questions. We'll also share case studies with Tokyu and X, highlighting their successful deployments of passkey authentication.
We'll close out the week with learning pathways for passkeys on Android and Chrome, insights from partners, and a new Compose sample app for Credential Manager with Android best practices and built using Compose.
We hope you'll join us in exploring these resources to learn how to elevate your app's security and user experience. We're excited to share this journey with you!
User safety is at the heart of everything we do at Google. Our mission to make technology helpful for everyone means building features that protect you while keeping your privacy top of mind. From Gmail’s defenses that stop more than 99.9% of spam, phishing and malware, to Google Messages’ advanced security that protects users from 2 billion suspicious messages a month and beyond, we're constantly developing and expanding protection features that help keep you safe.
We're introducing two new real-time protection features that enhance your safety, all while safeguarding your privacy: Scam Detection in Phone by Google to protect you from scams and fraud, and Google Play Protect live threat detection with real-time alerts to protect you from malware and dangerous apps.
These new security features are available first on Pixel, and are coming soon to more Android devices.
Scammers steal over $1 trillion dollars a year from people, and phone calls are their favorite way to do it. Even more alarming, scam calls are evolving, becoming increasingly more sophisticated, damaging and harder to identify. That’s why we’re using the best of Google AI to identify and stop scams before they can do harm with Scam Detection.
Real-time protection, built with your privacy in mind.
We’re now rolling out Scam Detection to English-speaking Phone by Google public beta users in the U.S. with a Pixel 6 or newer device.
To provide feedback on your experience, please click on Phone by Google App -> Menu -> Help & Feedback -> Send Feedback. We look forward to learning from this beta and your feedback, and we’ll share more about Scam Detection in the months ahead.
Google Play Protect works non-stop to protect you in real-time from malware and unsafe apps. Play Protect analyzes behavioral signals related to the use of sensitive permissions and interactions with other apps and services.
With live threat detection, if a harmful app is found, you'll now receive a real-time alert, allowing you to take immediate action to protect your device. By looking at actual activity patterns of apps, live threat detection can now find malicious apps that try extra hard to hide their behavior or lie dormant for a time before engaging in suspicious activity.
At launch, live threat detection will focus on stalkerware, code that may collect personal or sensitive data for monitoring purposes without user consent, and we will explore expanding its detection to other types of harmful apps in the future. All of this protection happens on your device in a privacy preserving way through Private Compute Core, which allows us to protect users without collecting data.
Live threat detection with real-time alerts in Google Play Protect are now available on Pixel 6+ devices and will be coming to additional phone makers in the coming months.
Posted by Neville Sicard-Gregory – Senior Product Manager, Android Studio
Looking for a more stable, reliable, and performant Emulator? Download the latest version of Android Studio or ensure your Emulator is up to date in the SDK Manager.
We know how critical the stability, reliability, and performance of the Android Emulator is to your everyday work as an Android developer. After listening to valuable feedback about stability, reliability, and performance, the Android Studio team took a step back from large feature work on the Android Emulator for six months and started an initiative called Project Quartz. This initiative was made up of several workstreams aimed at reducing crashes, speeding up startup time, closing out bugs, and setting up better ways to detect and prevent issues in the future.
A key goal of Project Quartz aimed to reduce Emulator crashes, which can frustrate and block developers, decreasing their productivity. We focused on fixing issues causing backend and UI crashes and freezes, updated the UI framework, updated our hypervisor framework, and our graphics libraries, and eliminated tech debt. This included:
As a result, we have seen 30% fewer crashes in the latest stable version of Android Studio, as reported by developers who have opted-in to sharing crash details with us. Along with additional end-to-end testing, this means a more stable, reliable, and higher quality experience with fewer interruptions while using the Android Emulator to test your apps.
This chart illustrates the reduction in reported crashes by stable versions of the Android Emulator (newer versions are at the top and shorter is better).
We have also enhanced our opt-in telemetry and logging to better understand and identify the root causes of crashes, and added more testing to our pre-launch release process to improve our ability to detect potential issues prior to release.
We also implemented several measures to improve release quality, including increasing the number and frequency of end-to-end, automated, and integration tests on macOS, Microsoft Windows, and Linux. Now, more than 1,100 end-to-end tests are ran in postsubmit, up from 500 tests in the past implementation, on all supported operating system platforms . These tests cover various scenarios, including (among other features) different Android Emulator snapshot configurations, diverse graphics card considerations , networking and Bluetooth functionality, and performance benchmarks between Android Emulator system image versions.
This comprehensive testing ensures these critical components function correctly and translates to a more reliable testing environment for developers. As a result, Android app developers can accurately assess their app's behavior in a wider range of scenarios.
It was also important for us to reduce the number of open issues and bugs logged for the Android Emulator by addressing their root cause and ensuring we cover more of the use cases you run into in production. During Project Quartz, we reduced our open issues by 43.5% from 4,605 to 2,605. 17% of these were actively fixed during Quartz and the remaining were closed as either obsoleted or previously fixed (e.g. in an earlier version of the Android Emulator) or duplicates of other issues.
While these improvements are exciting, it's not the end. We will continue to build on the quality improvements from Project Quartz to further enhance the Android Emulator experience for Android app developers.
As always, your feedback has and continues to be invaluable in helping us make the Android Emulator and Android Studio more robust and effective for your development needs. Sharing your metrics and crashdumps is crucial in helping us understand what specifically causes your crashes so we can prioritize fixes.
You can opt-in by going to Settings, then Appearance and Behavior, then System Settings, then Data Sharing, and selecting the checkbox marked ‘Send usage statistics to Google.'
Be sure to download the latest version of the Android Emulator alongside Android Studio to experience these improvements.
As always, your feedback is important to us – check known issues, report bugs, suggest improvements, and be part of our vibrant community on LinkedIn, Medium, YouTube, or X. Together, we can create incredible Android experiences for users worldwide!
We know how complex it can be to navigate the ever-changing landscape of commerce and payments, especially when it comes to global tax and regulatory compliance. In just two years, we've seen a significant increase in the number of new regulations impacting Google Play developers.
By partnering with Google Play, you're not just accessing a global marketplace serving over 190 countries; you're joining a powerful ecosystem built on security and trust. We understand the challenges these regulatory changes present, and we're here to support your growth every step of the way. That's why at Google Play, our teams work tirelessly behind the scenes to make compliance easier for you, providing a safe, trusted, and thriving marketplace for you and your users.
We're committed to investing in a seamless and efficient experience for developers on Google Play. Our platform helps you grow your business; here's how:
We're dedicated to supporting your growth in an ever-changing regulatory landscape and are constantly working to make Google Play the best platform for developers to thrive. Stay tuned for updates on new features, tools, and resources designed to help you grow your business and navigate the evolving apps and games landscape.
Tired of headaches with passwords? Ready for a future where online authentication is both faster and more secure? Then mark your calendars for Passkeys Week, November 18-22! Passkeys are an easier and more secure alternative to passwords, and are increasingly becoming the industry standard. Google is proud to support passkeys across Chrome, Android and beyond.
Part of our Spotlight Weeks series, this is your opportunity to dive deep into the world of passkeys – the revolutionary technology poised to replace passwords for good. Whether you're an Android or web developer, a security researcher, or just curious about the future of online identity, this week has something for you.
What are passkeys? They're a new type of credential that are far more secure than passwords and much easier to use. Imagine logging in with your device lock you already have set up, such as a fingerprint scan or a face scan, instead of typing out a complex string of characters. No password is used or stored on a server that could be compromised, keeping you safe from phishing attacks. And since there's no password, there' s no arcane string of characters to remember. That's the power of passkeys.
We're showcasing content designed to enhance your developer experience and help you get started with adopting passkeys. These items will include:
Passkeys Spotlight Week will happen entirely online at web.dev, developer.chrome.com and developer.android.com, and across Android and Chrome's Developer’s social media channels:
Follow us for the latest updates, spread the word about Passkeys Spotlight Week, and use #PasskeysWeek on your favorite social media platforms to ask questions and share your passkeys projects with the community. Check the Android Identity developer page on Monday, November 18, 2024 to read our next blog post with full details!
Don't miss this opportunity to learn from the best and be part of the passwordless revolution. Join us for Passkeys Spotlight Week and help shape the future of online authentication!