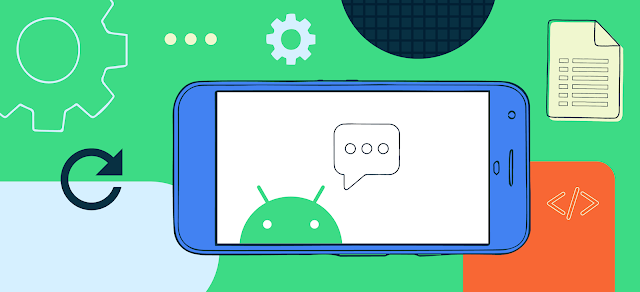
By Dan Albert, Software Engineer
One thing that NDK users struggle with is managing native dependencies:
- Library authors need to maintain support for both ndk-build and CMake (and hope that their users are using one of those two options and not something else).
- Libraries don’t always distribute prebuilt binaries for Android, so users must either build the library themselves or rely on (potentially untrustworthy) prebuilt binaries found elsewhere on the web.
- Android-specific build scripts are often out of date and no longer work.
- Libraries are sometimes built by a build system that Android doesn’t support.
- Libraries may not build on the user’s machine. For example, Unix shell scripts won’t run on Windows.
- Libraries often depend on other libraries, leaving users to chase them down and start the process again.
With version 4.0 of the Android Gradle Plugin, we’ve addressed these issues by adding support for distributing and exposing native libraries through the same mechanism that you do for Java libraries: Android Archives (AARs).
Here’s how you’d use curl and jsoncpp for example (and automatically pull in the implicit OpenSSL dependency that curl has):
// build.gradle dependencies { implementation 'com.android.ndk.thirdparty:curl:7.68.0-alpha-1' implementation 'com.android.ndk.thirdparty:jsoncpp:1.8.4-alpha-1' }
Note: With AGP 4.0 this is still experimental, so to enable this functionality you must set the following properties in your project's gradle.properties
file:
# Enables Prefab android.enablePrefab=true # Work around https://issuetracker.google.com/149575364 android.enableParallelJsonGen=false # 4.0.0 canary 9 defaults to Prefab 1.0.0-alpha3, which is not the latest. android.prefabVersion=1.0.0-alpha5
Importing packages into your build
Declaring the dependencies in your build.gradle
will cause Gradle to download those dependencies from Maven, but you must still instruct CMake or ndk-build how those dependencies should be used. Fortunately, the necessary CMake package config or ndk-build module will be automatically generated on your behalf. All you need to do is import and use them.
Here’s an example with CMake:
cmake_minimum_required(VERSION 3.6) project(app VERSION 1.0.0 LANGUAGES CXX) find_package(curl REQUIRED CONFIG) find_package(jsoncpp REQUIRED CONFIG) add_library(app SHARED app.cpp) target_link_libraries(app curl::curl jsoncpp::jsoncpp)
And here’s the same example with ndk-build:
LOCAL_PATH := $(call my-dir) include $(CLEAR_VARS) LOCAL_MODULE := libapp LOCAL_SRC_FILES := app.cpp LOCAL_SHARED_LIBRARIES := jsoncpp curl include $(BUILD_SHARED_LIBRARY) $(call import-module,prefab/curl) $(call import-module,prefab/jsoncpp)
And that’s it. In app.cpp
you can now do the following:
#include "curl/curl.h" #include "json/json.h"
A very common issue that people have is building OpenSSL to use with curl. While not explicitly mentioned in the build scripts above, the curl package itself depends on OpenSSL so this support is available automatically.
For the complete example, see the curl-ssl sample.
Prefab
The tool that facilitates all of this is called Prefab. Each AAR that exposes C++ libraries to its consumers packages their libraries, headers, and a small amount of metadata into the prefab
directory in the AAR. If the prefab
directory is found in an AAR dependency, the Android Gradle Plugin automatically runs Prefab to generate build system scripts from the contained information.
Each AAR might contain a large number of prebuilts for different configurations, so Prefab will perform a number of compatibility checks to find a suitable library for your build configuration. The selected library will match your build’s ABI, minSdkVersion
, STL choice, and be the best fit for the version of the NDK that you’re using.
What libraries are available?
We’ve already published the following libraries:
- com.android.ndk.thirdparty:curl:7.68.0-alpha-1
- com.android.ndk.thirdparty:jsoncpp:1.8.4-alpha-1
- com.android.ndk.thirdparty:openssl:1.1.1d-alpha-1
- com.google.oboe:oboe:1.3.0
For an up to date list, search https://maven.google.com/web/index.html for “com.android.ndk.thirdparty”.
How can I distribute my own libraries?
For the libraries we currently distribute, we wrote ndkports. This is a good fit if the library you’re building is a typical Linux or cross-platform project that doesn’t fit naturally into a typical Android build. If that’s a fit for the library you want, feel free to use ndkports for it, and consider sending us the patch!
If you’d like to request that Google maintain and publish an open source library in Prefab, use the “Package request” bug template on https://github.com/google/prefab/issues. Please keep in mind that each package does come with an ongoing cost, and we will be adding support on a limited basis so we will not be able to support everything.
Coming next is support for exposing your libraries in AARs using the existing Android Library publishing workflow.
Links
For more information about using native dependencies with the Android Gradle Plugin, see the documentation. For more examples, see the NDK samples.
If you’d like to learn more about Prefab itself, see its documentation on GitHub.
If you encounter any issues, file bugs in our Issue Tracker.