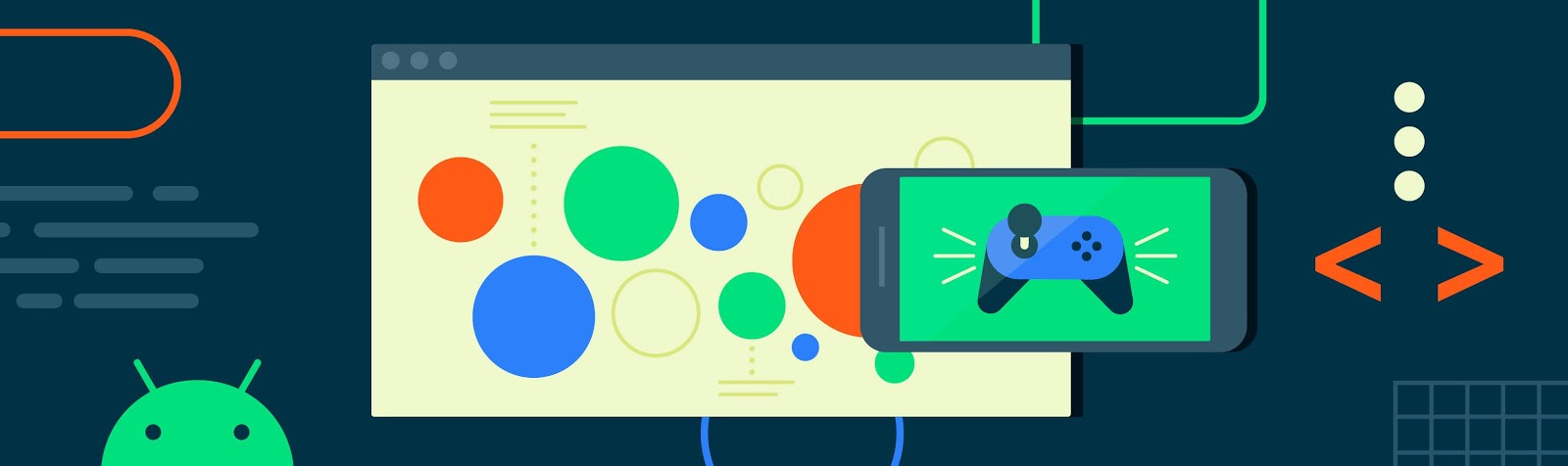
Android vitals is the destination for managing your app's technical quality. Over 80,000 developers take advantage of its performance and stability metrics every month.
As part of our work to help you deliver better game experiences to more Android users, we're introducing Android Performance Tuner - a new library in the Android Game SDK that unlocks game performance insights in Android Vitals. This gives you a scalable way to measure and optimize your frame rate and graphical fidelity across the whole Android device ecosystem.
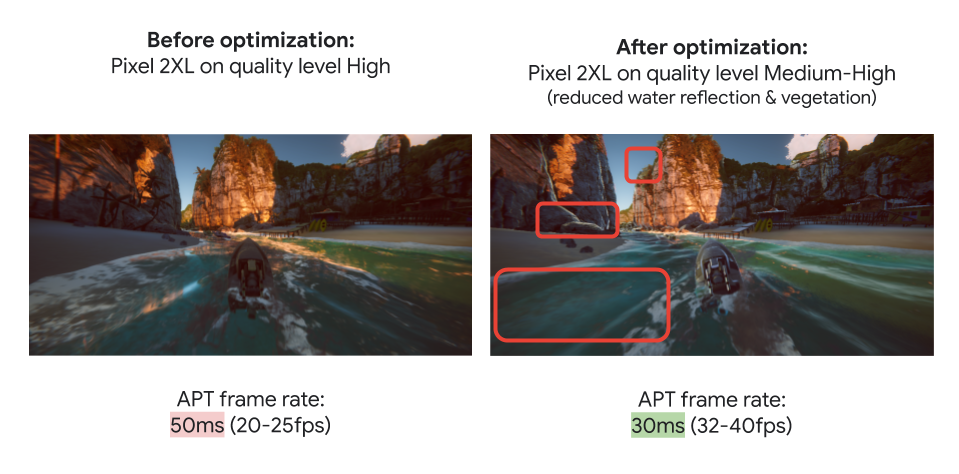
Unity Boat Attack Sample with Different Optimizations
Once you have integrated Android Performance Tuner into your game and published it on Play, you'll be able to see how it performs across real users and devices with the following new features in Android vitals.
Frame rate performance
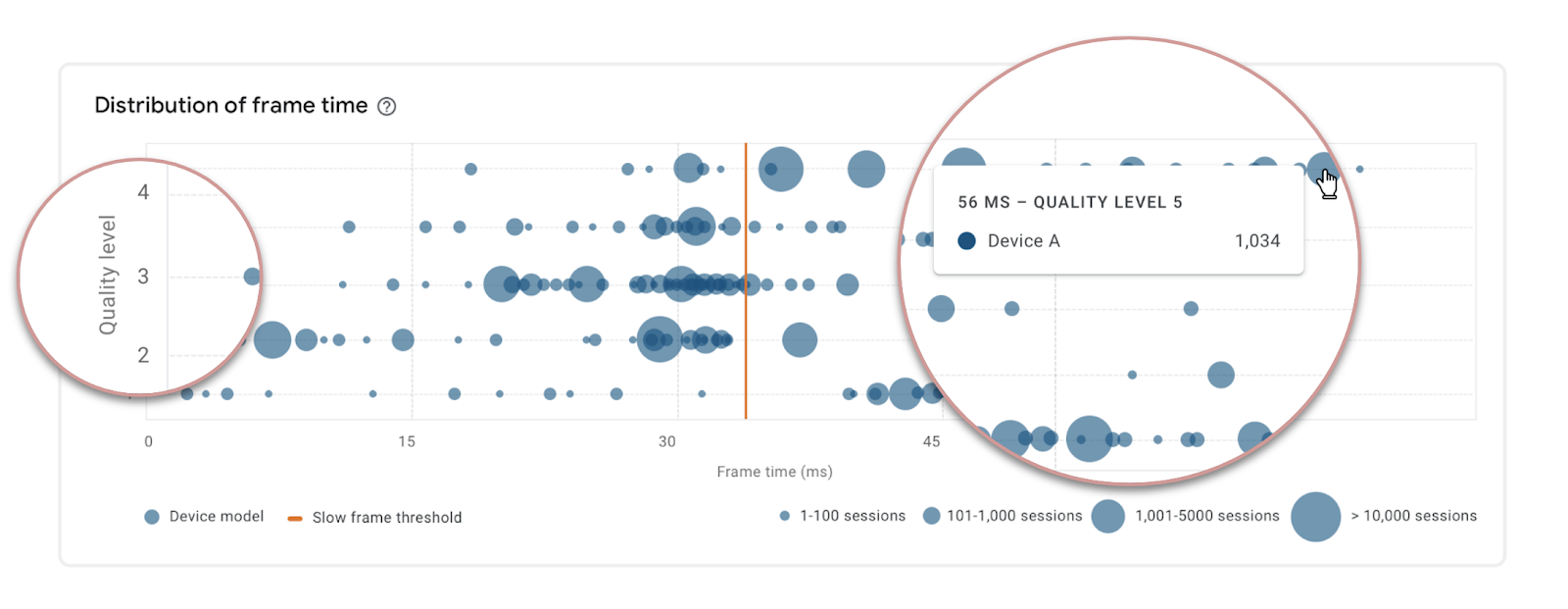
Frame Rate Performance by Quality Level and Device Model
We chart the frame time distribution across your users’ devices, broken down by quality levels that you have implemented in your game, so you can see how specific device models or hardware specifications are performing on each quality level.
Performance issues
We also analyze your performance data to help determine the likely cause of issues, so you can differentiate between problems associated with specific hardware and problems with specific screens or levels in your game. You annotate your code to give contextual information about what your game is doing at that point. This gives you full control over the granularity of the insights.
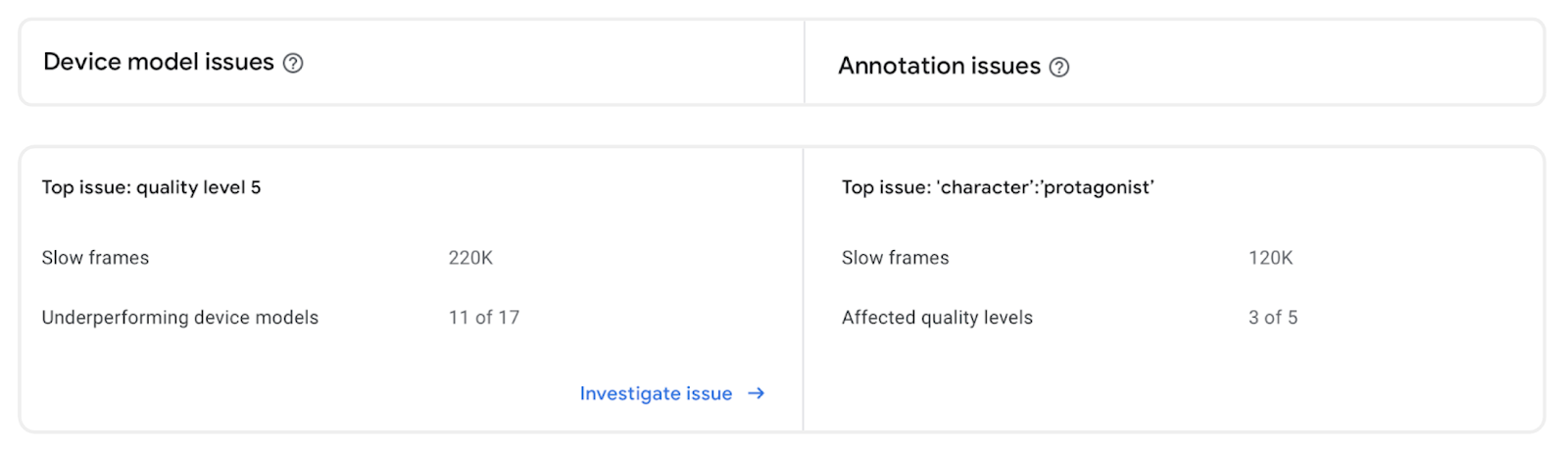
Top Device Model/Annotation Issues
We call out the top device model issue as well as the top game-specific issue to give you clear guidance on what's most important.
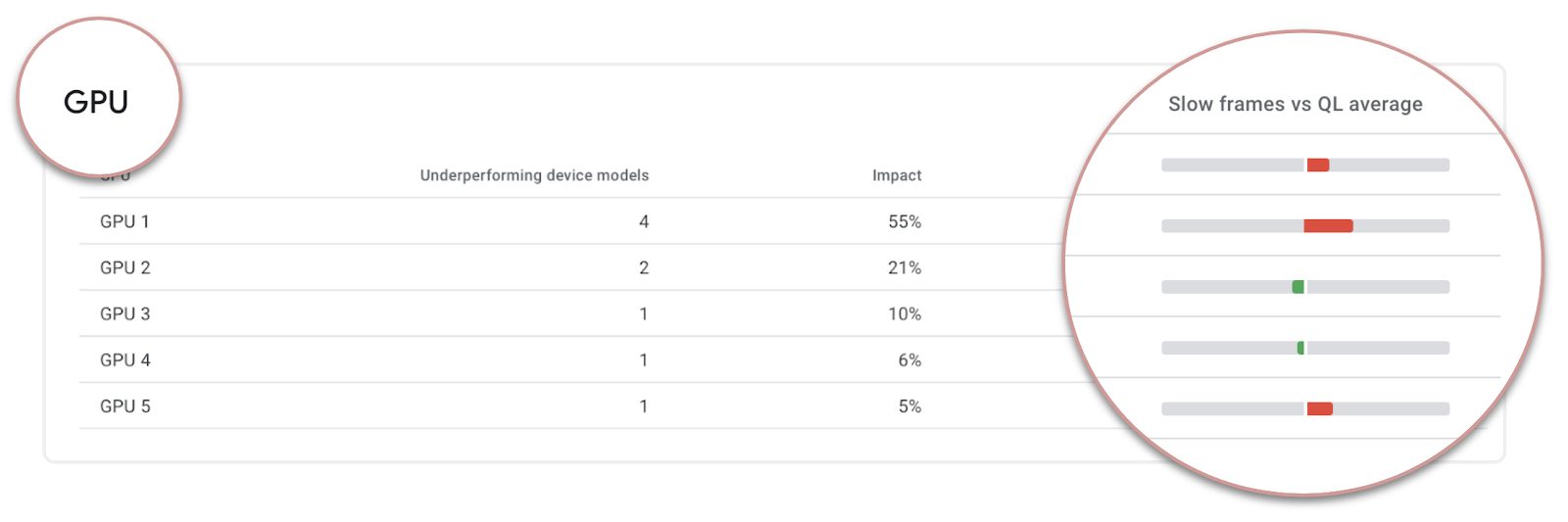
Underperforming Device Models by GPU
You can drill down to see a breakdown of underperforming device models by different specs, such as GPU and SoC. This allows you to decide whether you can work at the GPU or SoC-level to optimize performance. Alternatively, you may decide to change quality levels, rather than work at the device model level.
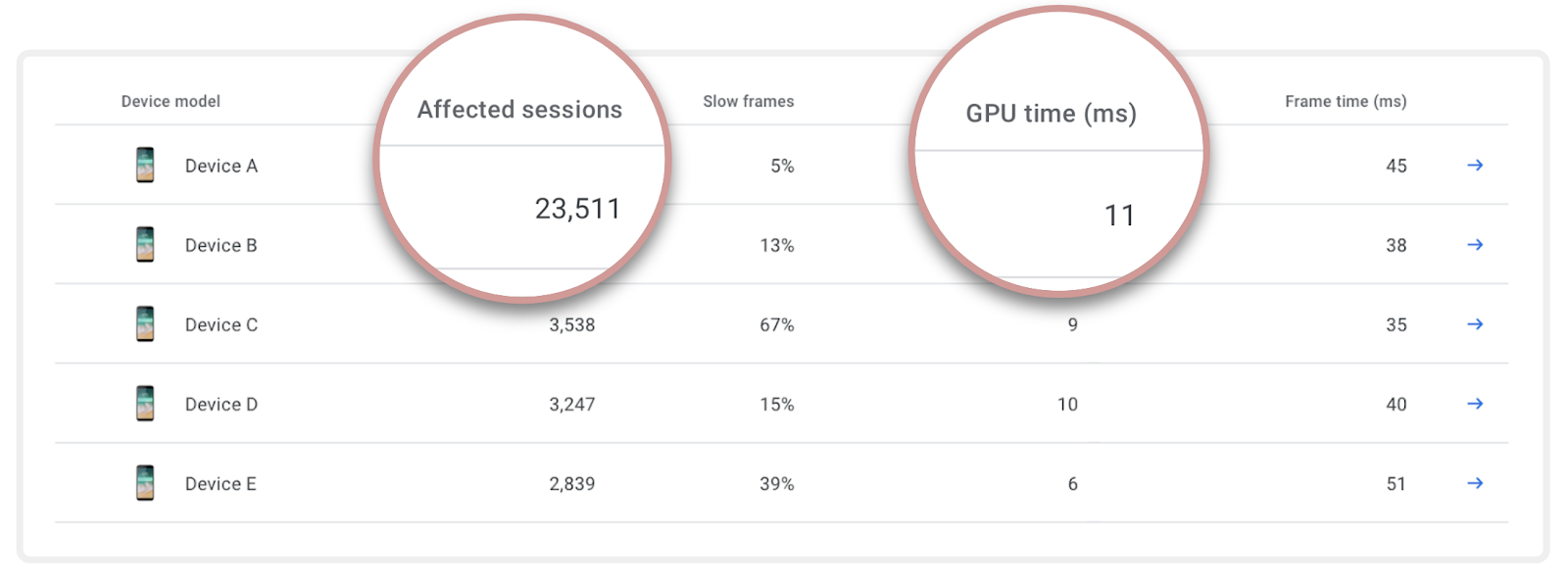
Device Model Impact, User Impact, GPU Time
You can also see the full list of device models, along with the number of affected user sessions and frame time, to help you prioritize device-specific changes. As well as total frame time, we also show you GPU time to help determine whether the device is GPU bound or has another performance problem, such as being CPU or I/O bound. All data in the device model table can be exported for further analysis and action planning.
Opportunities to make a good experience great
We can also help identify opportunities — places where you could potentially provide users with a better experience by giving them a higher quality level, enabling more advanced graphical features.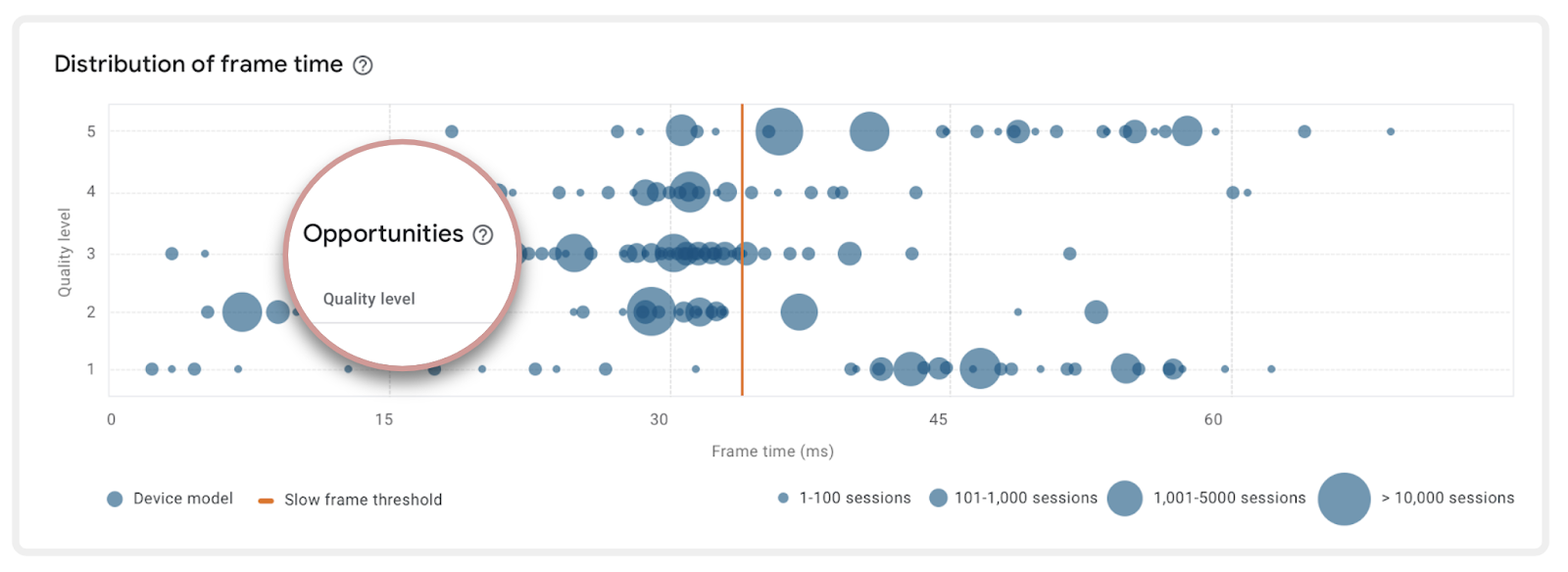
Frame Time Performance with Opportunities
The devices on the far left are more than meeting the frame times for smooth performance. You can drill down to see stats by device model and specification to see if there is an opportunity to improve the graphical fidelity across a wide range of devices.
Available (almost) everywhere
The Android Performance Tuner is intended to work across over 99% of the Android device ecosystem. You can get these insights on any Android devices around the world, from Android 4.1 (API 16) onwards.
Integrating Android Performance Tuner
Whether you have your own game engine or are using a third-party game engine, we're doing our best to make integration easy. The Android Performance Tuner relies on tick functions being called each frame. Within the library, this tick information is aggregated into histograms, which are periodically uploaded through an HTTP endpoint, so your game will need to have the internet access permission.
With our plugin for the Unity platform, you can collect frame ticks from Unity 2017.4 onwards. Unity 2019.3.14+ enables the collection of higher-fidelity performance information.
If you're doing a native source code level engine integration, we strongly recommend integrating the Frame Pacing API from the Android Game SDK to get the highest quality information. The Frame Pacing API will give you smoother frame rates and improved support for high-refresh rate displays, so it's worth integrating on its own.
Unreal 4.25+ integrates the Frame Pacing API. You enable it by adding a.UseSwappyForFramePacing=1
to the Android_Default profile to activate it for all Android devices.
Within Unreal or your native engine integration, you pass in the Swappy_injectTracer
function from the Frame Pacing API at initialization to enable automatic frame time recording.
void InitTf(JNIEnv* env, jobject activity) { SwappyGL_init(env, activity); swappy_enabled = SwappyGL_isEnabled(); TFSettings settings {}; if (swappy_enabled) { settings.swappy_tracer_fn = &SwappyGL_injectTracer; } … }
Enabling Frame Time Recording in the Android Performance Tuner for your Engine
In Unity, we recommend activating Optimized Frame Pacing within the Unity settings (Unity 2019.3+ only), but Frame Pacing isn't required in Unity to use the Android Performance Tuner.
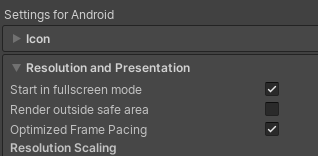
Activating Optimized Frame Pacing in Unity 2019.3
Providing contextual information
Next, you want to define annotations to give contextual information about what your game is doing when a tick is recorded, such as:
- Current game level
- Loading a specific scene
- A "big boss" or other complex rendered item is on the screen
- Relevant game state information
Annotation Parameters
If you're using Unity with the Android Performance Tuner plugin, you'll automatically get a scene annotation that maps to the current scene being played. The LoadingState annotation can be easily hooked up to your scripts, and you can define additional annotations within the plugin editor UI.
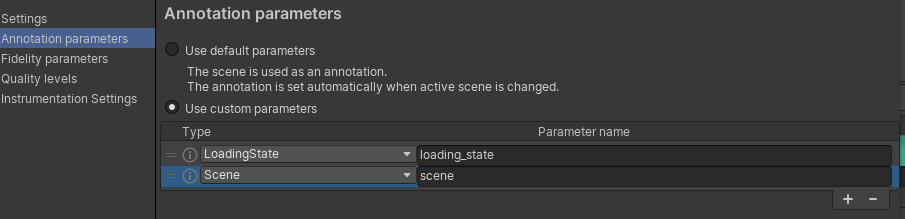
Annotation Parameters within the Unity Editor from the Android Performance Tuner Plugin
To pass annotation parameters from within your own game engine, you define a protocol buffer message that contains all of these annotations, such as loading state, level or scene, etc.
Fidelity Parameters and Quality Levels
You also define fidelity parameters and associate them with quality levels that your game reports back. These can be used for anything that you use in your game to reduce the complexity of the scene, such as texture quality, draw distance, particle count, post-processing effects, shadow resolution, etc. In the native integration, you define these parameters using a protocol buffer.
import "tuningfork.proto" message FidelityParams { int32 texture_quality_level = 1; int32 shadow_resolution = 2; float terrain_details_percent = 3; int32 post_processing_effects_level = 4; }
Example FidelityParams Proto Definition for an In-house Engine
Then, you create up to fifteen sets of quality levels as a set of values defined by the FidelityParams message, which allows the Android Performance Tuner to track its metrics against your quality data. You can create both fidelity parameters and quality levels in the Unity editor interface provided by the Android Performance Tuner for Unity plugin.
Testing your integration
We've created the Tuning Fork Monitor app to act as a local server and display data from an Android Performance Tuner-enabled app. You can call EnableLocalEndpoint()
in the Android Performance Tuner Unity plugin on a development build to enable local testing. In your native integration, you set the endpoint_uri_override
in the Android Performance Tuner settings.
Once local tests look great, you then enable the Android Performance Parameters API in the Google Cloud Console to test end-to-end.
Available Now
We're committed to helping you bring the best version of your game to the widest number of users and devices in the Android ecosystem. Android Performance Tuner within the Android Game SDK, the Unity plugin, and Performance Insights within Android Vitals are all available now. You can refer to our documentation for a walk through of the process for native and Unity integrations.