Tech on the Toilet (TotT) is a weekly one-page publication about software development that is posted in bathrooms in Google offices worldwide. At Google, TotT is a trusted source for high quality technical content and software engineering best practices. TotT episodes relevant outside Google are posted to this blog.
We have been posting TotT to this blog since 2007. We're excited to announce that Testing on the Toilet has been renamed to Tech on the Toilet. TotT originally covered only software testing topics, but for many years has been covering any topics relevant to software development, such as coding practices, machine learning, web development, and more.
A Cultural Institution
TotT is a grassroots effort with a mission to deliver easily-digestable one-pagers on software development to engineers in the most unexpected of places: bathroom stalls! But TotT is more than just bathroom reading -- it's a movement. Driven by a team of 20-percent volunteers, TotT empowers Google employees to learn and grow, fostering a culture of excellence within the Google engineering community.
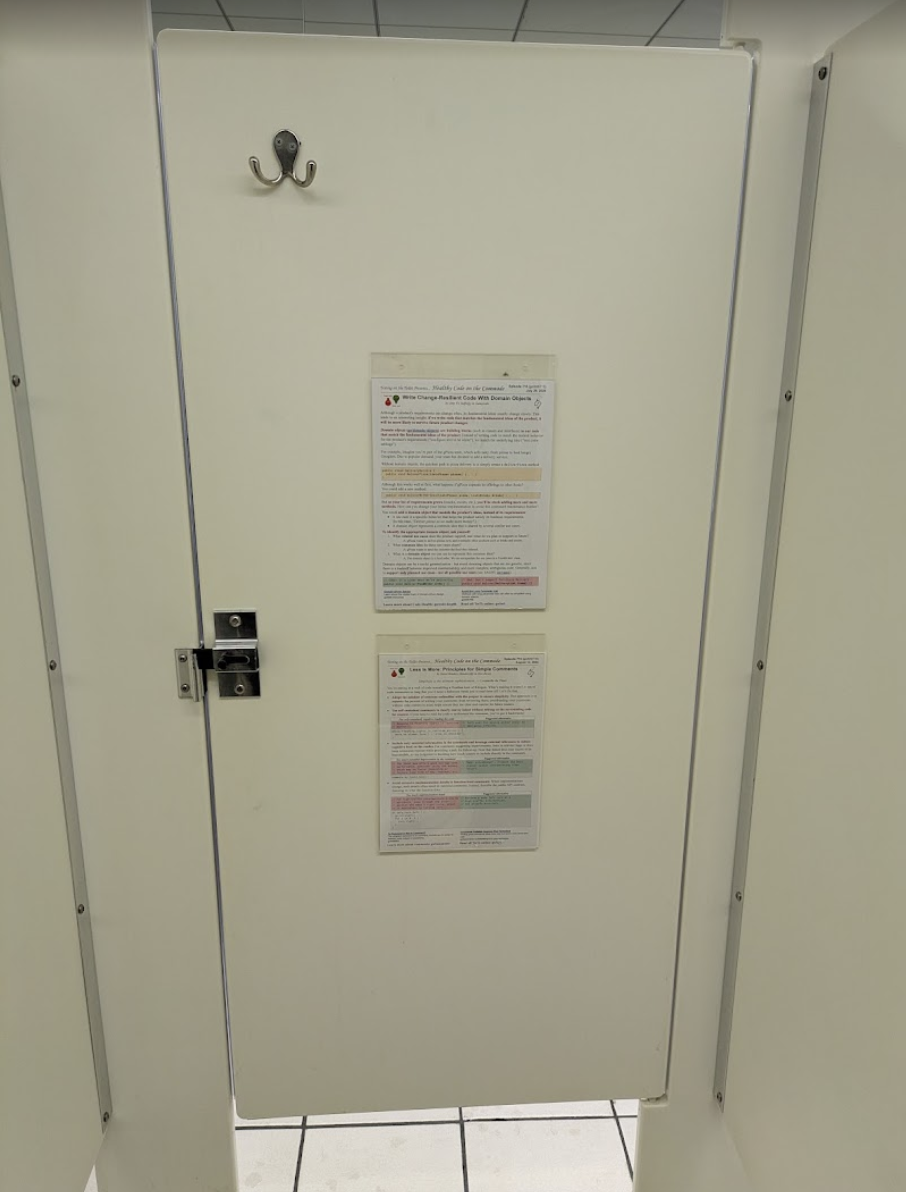

Photos of TotT posted in bathroom stalls at Google.
Anyone at Google can author a TotT episode (regardless of tenure or seniority). Each episode is carefully curated and edited to provide concise, actionable, authoritative information about software best practices and developer tools. After an episode is published, it is posted to Google bathrooms around the world, and is also available to read online internally at Google. TotT episodes often become a canonical source for helping far-flung teams standardize their software development tools and practices.
Because Every Superhero Has An Origin Story
TotT began as a bottom-up approach to drive a culture change. The year was 2006 and Google was experiencing rapid growth and huge challenges: there were many costly bugs and rolled-back releases. A small group of engineers, members of the so-called Testing Grouplet, passionate about testing, brainstormed about how to instill a culture of software testing at Google. In a moment of levity, someone suggested posting flyers in restrooms (since people have time to read there, clearly!). The Testing Grouplet named their new publication Testing on the Toilet. TotT’s red lightbub, green lightbulb logo–displayed at the top of the page of each printed flyer–was adapted from the Testing Grouplet’s logo.
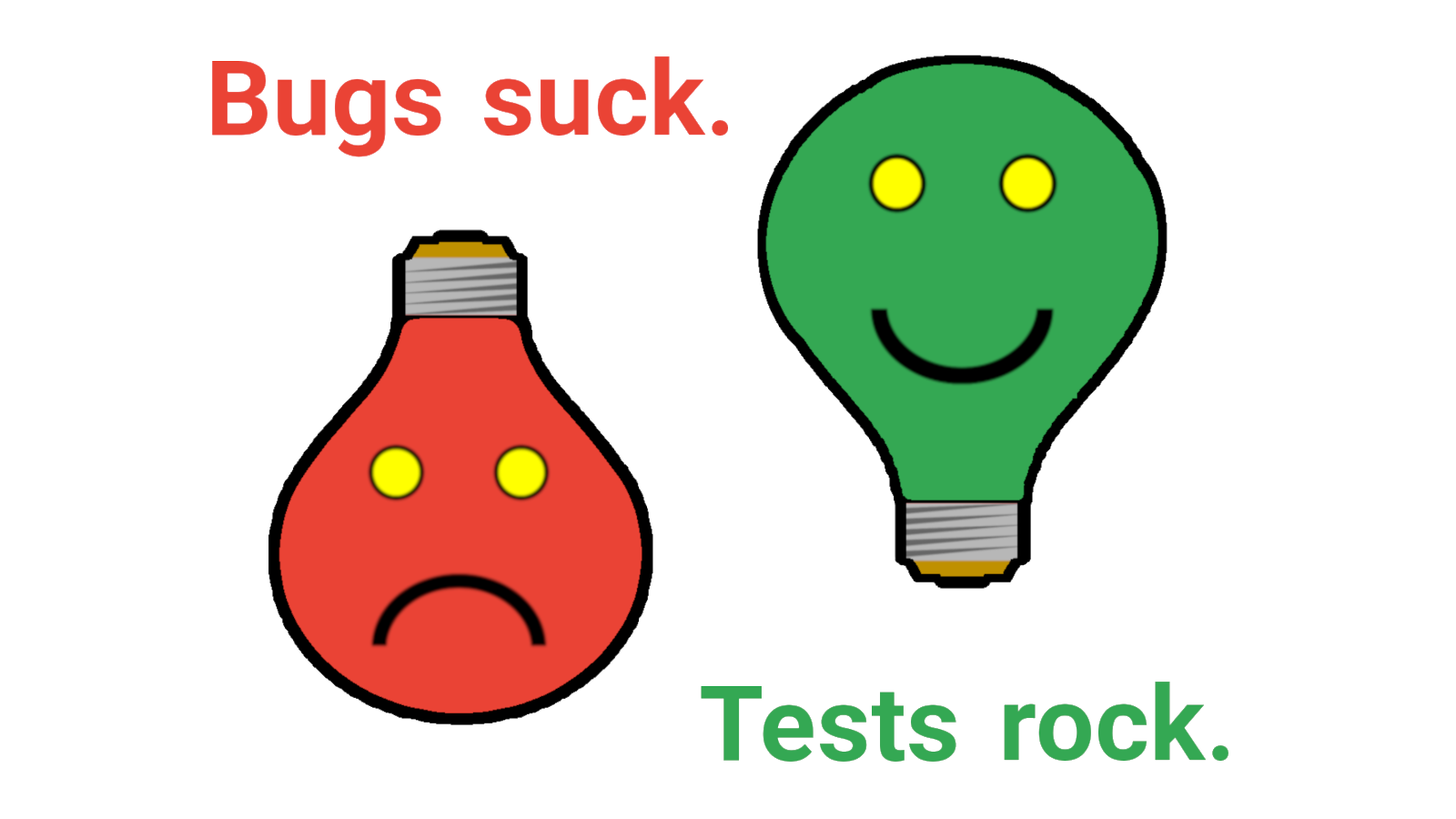
The TotT logo.
The first TotT episode, a simple code example with a suggested improvement, was written by an engineer at Google headquarters in Mountain View, and posted by a volunteer in Google bathrooms in London. Soon other engineers wrote episodes, and an army of volunteers started posting those episodes at their sites. Hundreds of engineers started encountering TotT episodes.
The initial response was a mix of surprise and intrigue, with some engineers even expressing outrage at the "violation" of their bathroom sanctuary. However, the majority of feedback was positive, with many appreciating the readily accessible knowledge. Learn more about the history of TotT in this blog post by one of the original members of the Testing Grouplet.
Trusted, Concise, Actionable
TotT has become an authoritative source for software development best practices at Google. Many episodes, like the following popular episodes at Google, are cited hundreds of times in code reviews and other internal documents:
A 2019 research paper presented at the International Conference of Software Engineering even analyzed the impact of TotT episodes on the adoption of internal tools and infrastructure, demonstrating its effectiveness in driving positive change.
TotT has inspired various other publications at Google, like Learning on the Loo: non-technical articles to improve efficiency, reduce stress and improve work satisfaction. Other companies have been inspired to create their own bathroom publications, thanks to TotT. So the next time you find yourself reading a TotT episode, take a moment to appreciate its humble bathroom beginnings. After all, where better to ponder the mysteries of the code than in a place of quiet contemplation?